Workbook exercise #4: Second VBA exercise for Word
Due dates for all assignments can be found on the [main
grid] of the course webpage.
Note: The computer lab for CPSC 203 is located in SS018 (the "Tri-Labs").
If you find that your particular version of Word (e.g. Office 365, older
versions of Office, MAC users) does not include everything needed for a feature
then you should to complete that feature in SS018. Alternatively for the remote
learning version of the course you can login to a lab computer from your own. [Information
link created by UC-IT with modifications by James Tam, for questions:
www.ucalgary.ca/it]
Introduction and tip for programming
This
assignment will be more challenging than the other workbook exercise. Partly
it's because the first exercise was simply to show you exactly what you need to
do create and run a working
VBA program, partly because this exercise is give you some actual practice writing a
program that requires more than just following a bunch of steps or looking up
the answer in the notes. In that way it's similar to the full assignment coming
up you must think through your solution, albeit the solution for this exercise is far less complex,
but it will help you prepare for writing the larger and more complex
program needed for the next assignment. And it will provide you with a more
realistic idea of what programming is like than the first exercise. Actual
programmers don't just follow a sequence of prescribed steps or "look up an
answer somewhere" when creating a computer program. Instead it requires that
they understand basic programming concepts (e.g. getting user input, displaying
output, using variables, branching etc.) and figuring out how to apply those
concepts with the program that they must create. In a way it's analogous to
creative writing. You first learn the grammar of the language. Next you study
the elements associated with good writing style. Then you must determine how to
apply what you have learned in order to tell a good story well - there isn't an
exact sequence of steps you can apply for every situation nor can you simply
lookup parts of your story someplace. Also, similar to creative writing, one
gets better by 'doing'.
In the case
of programming the doing means writing programs (from scratch) on your own.
Examples of practice problems include: the ones listed in the course workbook,
example problems covered in tutorial and lecture, suggested problems that may be
described in lecture. If you have time then you can even try writing the example
problems from lecture and tutorial on your own. Don't just look at the final
solution! You will get more out of this process if you write a program from
scratch. That program should implements the same features as the example we
covered. You should do this without looking at the solution because that's just
copying an answer. However you can look at the course notes and other examples
to remind you of how things work in VBA.
Description
Here is a [starting
document]
to ensure that you at least start with the type of Word document that can
contain a macro. (It doesn't contain any VBA instructions). The document is
provided so you start with a Word document that can contain a macro (docm), whether or not you use it is entirely
up to you. If you are unsure of what is required in the creation of a VBA
program then you should refer the extra notes that you should have been taking
while I covered the material to
the first set of VBA lecture notes.
Below is one
important reminder image of how you should save your macros (in this case the
name of the Word document to contain the VBA macro is called "starting document"
but your program (and the Word document that contains it) must be called "exercise4VBA").
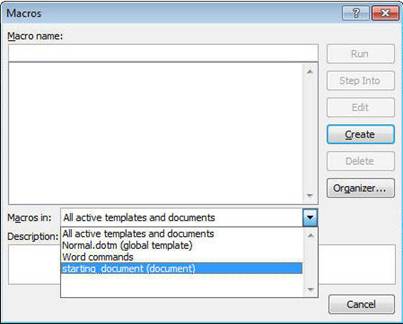
Because
your macros will be included in the document, when it comes time to submit your
work you can just upload the macro-enabled document. Double check that your
VBA macro really is in the Word document that you upload! It is up to you to
do the check, don't rely on us to do it for you. If you want to be
extra safe (and I highly recommend that you do this) you can copy paste your VBA
program into a regular Word document and submit that extra Word document as well
the macro-enabled document. Alternatively
you can just copy-paste your VBA program from the Visual
Basic editor into the
exercise4VBA
Word document.
It would be a good idea to check your submission by downloading what you
uploaded into D2L and actually open that document, ideally on a different
computer that the one you used to write your VBA program (to help ensure that
you aren't looking at a local file on the first computer which you used to write
the program). As was the case with the assignments do not use other compression
utilities (such as zip), otherwise your submission may not be marked.
Requirements for this exercise
Similar to
the assignment everyone starts out with a grade point of zero for this exercise.
As you implement the features listed below your grade will increase.
Write a VBA
program called "exercise4VBA".
Again: that should also be the name of your Word document as well.
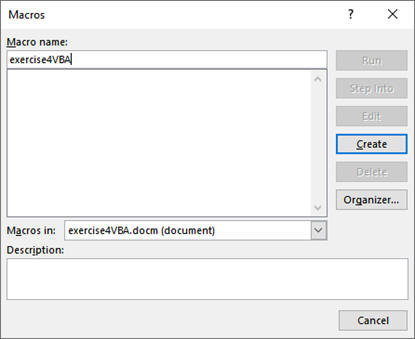
To help you test
your program there are 4 Word documents. Download these to folder that contains
the VBA program that you are creating for this exercise. In addition to testing
your program with these documents you should test your solution with test cases
in other Word documents that you have made yourself to check any other cases
that you might think are relevant.
You don't
need to submit these 4 Word documents. The marker will have access to these
documents to test your submission and may perform additional tests with other
documents. But you should test your program on your own with
these files because the program you need to submit will have to handle the
required cases properly.
Program
features and grading.
-
The following two popup messages will always appear: This is
regardless of the number of typographical mistakes and inline shapes that
reside in the document.
The counts will carried out via the 'ActiveDocument'
object.
- The program will count and display using a MsgBox (0.5 GPA) the number of typo graphical errors. The message must take the
form
<"Num typos: "> <Actual # typographical mistakes>.
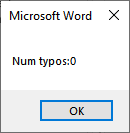
- Count and display using a MsgBox the number of 'InlineShapes' which is (0.5 GPA) in the document.
The message must take the form
<"Num inline shapes: ">
<# of inline
shapes>.
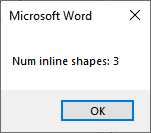
- To make an open Word document active you can click on it.
- For instance, in order to test your program
with the document for Case 1A you would open the document
'case1A_has_typo_zero_shapes' (or click on the window containing
document if it is already open). Then without clicking on any other
Word documents (not even the one containing your VBA program) you would
go into the VB editor and run your program. Because the last Word
document that you clicked on was case1A_has_typo_zero_shapes then that
is the Word document where the count of spelling mistakes and number of
inline images would be performed. [Video showing
how to make the Case 1A document the active one] Notice in the video
how I don't run the program by clicking on the Word document containing
the VBA program but instead I click on the play icon in the Visual basic
editor. If I ran the program by clicking on the VBA macro enabled
document then the macro enabled document would be the active one and not
the desired file 'case1A_has_typo_zero_shapes'.
Required cases: The content of
the popups will vary depending upon the number of typographical mistakes and
the inline shapes in the test document.
- After getting the counts the program will display one of 3 MsgBox popups depending upon the following
conditions. You need to use IF structures
in order to ensure that the correct message appears under the correct condition.
- Condition 1 (1.0 GPA):
The currently active Word document has one or more spelling mistakes (as
counted by your VBA program) the popup message will take the form <"Num typos: "> <Actual # typographical mistakes>
<", Num inline shapes: not counted">. As
you can see the number
of inline shapes will have no bearing on whether or not this popup appear i.e.
the number of inline shapes is irrelevant if there any typographical
errors in the document.
-
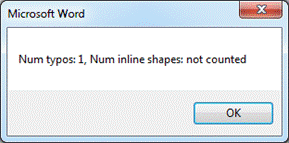
- Condition 2 (1.0 GPA):
The currently active Word document has no spelling mistakes (as
counted by your VBA program) and there are less than 3 inline shapes the popup message will
take the form <"Num typos:
"> <Actual # typographical mistakes> <", Num inline shapes: too low">.
-
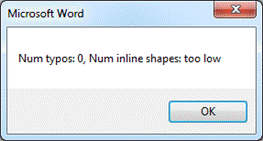
- Condition 3 (1.0 GPA):
The currently active Word document has no spelling mistakes (as
counted by your VBA program) and there are at least 3 inline shapes the popup message will
take the form <"Num typos:
"> <# typographical mistakes> <", Num inline shapes: "> <# of inline
shapes>.
-
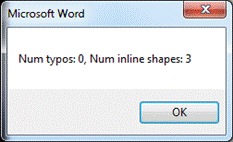
In terms of the output of the message box for the 3 conditions: the
part between the quotes is what literally appears in the popup, the part
that is italicized and not quoted will be a number that varies depending
upon the number of spelling mistakes or the number of inline shapes in
the document. You will only get credit for the above 3 popups if
the messages appear under the correct conditions (described under
each of the 3 conditions).
Documentation requirements
Although you won't be graded on documentation for exercises it still would be
a good idea to include it so you don't miss it in the assignment. What's needed
for assignments include: your full name as provided to the university (make sure
it matches, don't include 'nicknames'), student identification number, tutorial
number. Also you should clearly specify what features of the assignment that you
completed or didn't complete. In order to get credit the documentation has to be
clear and complete
Similar to documentation requirements you aren't graded for style for workbook
exercises but it would be a good idea to still follow those requirements so you
get in the habit of doing this.
- Each level of code indenting is
consistently 1 tab. Instructions
in the sub-routine (between the 'sub'
and 'end-sub' is 1
level, the body of IF or WHILE structures
counts as another level of indenting. An IF within
an IF or WHILE within
a WHILE (or even
an IF within a WHILE or
a WHILE within an IF) each
count as another level.
- Good naming conventions (e.g. variables,
sub-routines, the name of Word document containing the VBA program and
constants if applicable) are followed. Some examples of naming conventions
are provided in [the VBA Part I
notes].
-
Operating system specific issues:
submissions must
work on the machines a Windows computers. (For the remote learning
semester since access to the lab computers is more challenging: the
requirement is that your document works on any Windows computer). It's up to
you to test and check this is the case. Non-functional submissions will
receive only partial credit (if any at all).
Although operating system issues are rare (non-existent?) if you implement
your solution on another operating system it's a good idea to check your
submission on a Windows computer. (See the administrative video lecture
loaded into D2L for the week of Jan. 9 - 15 for suggestions as to how you can do this).
Caution for Windows users: take care that you
don't accidentally submit a shortcut to a file instead of the actual file.
(Check the file name and compare the file size to your original file. Simply
downloading the shortcut file as a test won't work because that shortcut
will work on your computer but not on anyone else's machine).
-
Late assignments or
components of assignments: Extensions may
be granted for reasonable cases by the course instructor with the receipt of
the appropriate documentation (e.g., a
statutory declaration with a commissioner of oaths). Location of the
online form: [PDF
file]. Alternate submission mechanisms (non exhaustive list of examples:
email, uploads to cloud-based systems such as Google drive, time-stamps, TA
memories) cannot be used as alternatives if you have forgotten to submit
work or otherwise have not properly submitted into D2L. Only files
submitted into D2L by the due date is what will be marked,
everything else will be awarded no credit. The final cut off date after
which full assignments will not be accepted is after the maximum
progressive penalty (listed below) can be applied.
-
Assignment extensions (must
be requested before the assignment is due): may be granted for reasonable
cases by the course instructor with the receipt of the appropriate
documentation (e.g.,
a sworn
declaration signed by a commissioner of oaths). Typical examples of reasonable
cases for an extension include: illness or a death in the family. Example
cases where extensions will not be granted include situations that
are typical of student life: having multiple due dates, work commitments,
forgetting or mixing up due date etc. Tutorial instructors (TAs) will not be
able to provide extension on their own and must receive permission from the
course instructor first. If
you request an extension from me let me know the name of your tutorial
instructor and the tutorial number [link to :
tutorial and TA information) because the markers won't accept
late submissions without directly getting an email from me and I need to
know who to contact. Also extensions need to be requested before the
due date and not afterward.
-
How you will be graded for assignments. Because
most exercises are fairly simple a marking spreadsheet isn't required.
Instead you can find your grade point in the D2L Dropbox and read any
comments that the Teaching assistant may have entered.
-
Questions or
concerns about grades have been released: Assignments will be marked by
your tutorial instructor (the "Teaching Assistant" or "TA") for your tutorial
section (instructor information listed here as well). When you have questions about marking
this is the first person
that you should be directing your questions towards. If you still have
question after you have talked to your TA, then you can talk to your course
(lecture) instructor but please indicate in your email that you first
contacted your TA before going into your concerns.
Collaboration:
Assignments
must reflect individual work;
group work is not allowed in this class nor can you copy the work of others.
Some "do nots" for your solution: don't publically
post it, don't email it out, don't show it to other students,
don't get help with your assignment from a tutor (if you have hired one).
For more detailed information as to what constitutes academic misconduct
(i.e., cheating) for this course please read the following [link].
Submitting your work:
-
The document must be electronically submitted using D2L.
-
You need to submit the correct Word document that contains the
macro (a macro-enabled Word document
.docm).
-
Make
sure that you [check
the contents of your submitted files]
(e.g., is the file okay or was it corrupted, is it the correct version etc.).
It's your responsibility to do this! (Make sure that you submit your
assignment with enough time before it comes due for you to do a check). If don't
check and there were problems with the submission then you should not expect
that you can "learn your lesson" and simply resubmit.
-
Do
not use compression utilities (such as zip) or archiving utilities (such as
tar) otherwise your submission may not be marked. The space savings in D2L
is not worth the extra time required by the marker to process each
submission.
-
How often can you submit:
Multiple submissions are allowed for this assignment: You can (and
really should) submit work as many times as you wish before the due
date. Due dates are strict, only what is in D2L by the deadline is what
will be marked.
Other methods of verifying that your work was completed
on time (e.g. checking timestamps, emailed files etc.) will NOT be
accepted. However only the
latest versions of each individual document are the ones that will be
marked, everything else will be ignored (because it is not fair to your
marker to sort through multiple versions of your files). In the case of
A1 the marker will just look at the latest version of: Calgary and the merged letter
that the marker can 'click through'.
Late
submissions for assignments when there is no extension granted: Make
sure you give yourself enough time to complete the submission process so
you don't get cut off by D2L's deadline (or your submission will be
automatically flagged as late by D2L and it will be graded appropriately)..
Submission received: |
On time |
Hours late : >0 and <=24 |
Hours
late: >24
and <=48 |
Hours
late: >48
and <=72 |
Hours
late: >72
and <=96 |
Hours
late: >96 |
Penalty: |
None |
-1 GPA |
-2 GPA |
-3 GPA |
-4 GPA |
No credit
(not accepted) |