CPSC 233: Assignment 4 (Worth 10%)
New concepts to be applied in this assignment
- Designing and implementing a moderately sized program
- Relationships between classes
- Message passing
Introduction
Background
It's hard to believe
but the 'Trek' franchise © has been
around for over 40 years. Production of the first episode "The
Cage" began in late 1964. It was given a budget of
$640,000 by NBC and produced by Desilu Studios (which was later taken over
by Paramount). Since that historic date, Star Trek has
become a part of television and motion picture history and includes six
TV series, ten motion pictures (with an
eleventh movie in the works) and at least two Trek-themed vacation spots:
Vulcan Alberta
and
Star Trek, the experience in Las Vegas. |
|
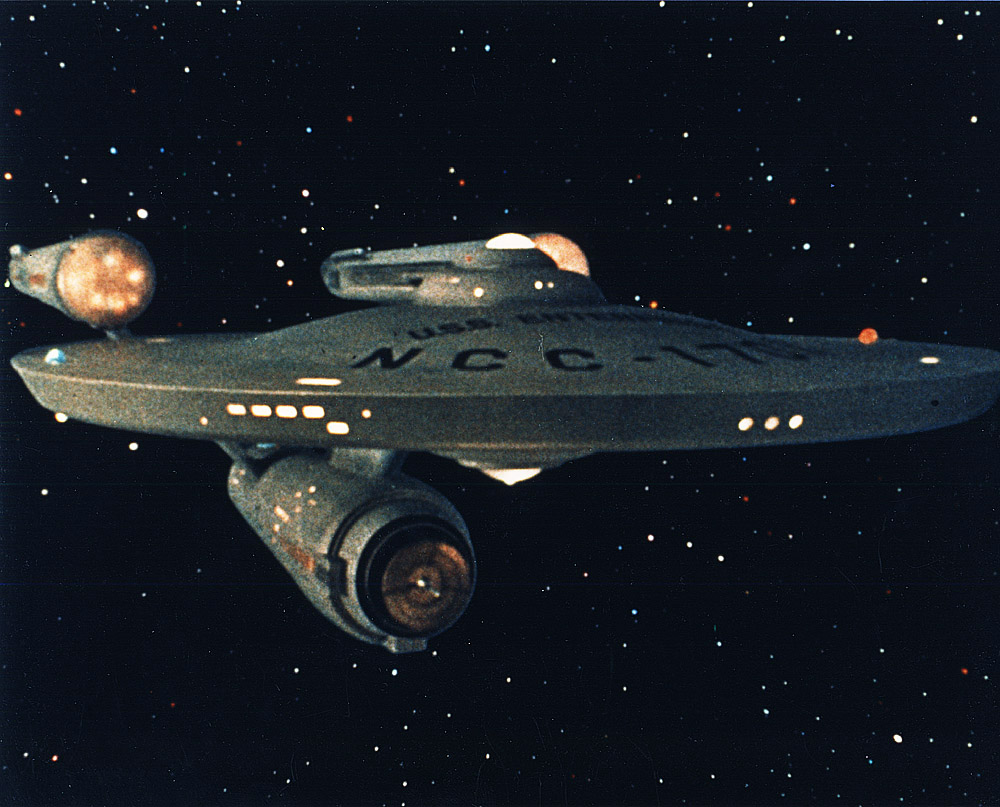 |
Your mission for this assignment
For this assignment you are to implement a
simple text based Star Trek game (Figure 1). The Trek galaxy (i.e., the quarter of
our galaxy known as the "Alpha quadrant") will be
modeled with a ten-by-ten two-dimensional array of references. Each array
element (called a "sector") will either be null (representing empty space) or
contain a reference to a 'Starship'. Starships will be represented
either by the 'H' character (which is the ship that is controlled by the human
player) or a 'C' (for the three computer-controlled ships). When the
game starts, the human player's starship will always start off in the same
location, the "Sol sector" (0,0). The
coordinates of the three computer-controlled ships will be randomly determined.
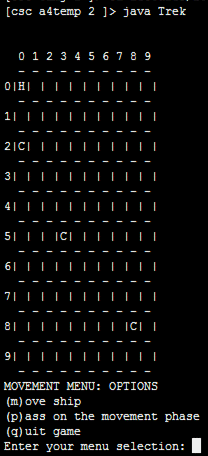 |
Figure 1: Your mission,
re-create the Trek universe. |
A quick introduction to the Trek universe
You don't have to be die-hard Trek fan to complete all the features of this
assignment (although you might enjoy it more if you were :-)). Here's a
brief introduction to the relevant parts so that you know how things work enough
to get started on the assignment.
Starships are equipped with a
faster-than-light engines known as "warp drive". In terms of the game
a ship can move into one of the adjacent sectors (Figure 2). Even with warp engines a ship still cannot leave the
bounds of the galaxy (i.e., exceed the bounds of the array).
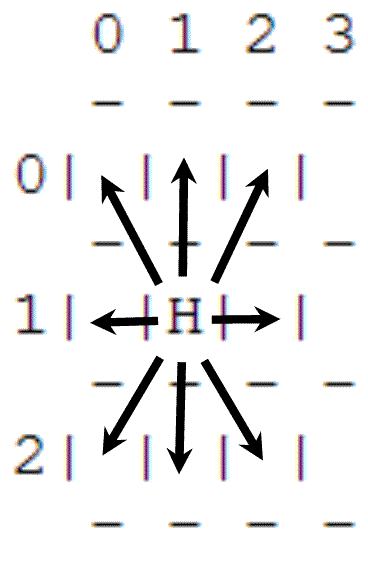 |
Figure 2: Warp
drive can take a ship to any adjacent sector |
Each starship is equipped with a powerful
(~1 Gigawatt) energy beam weapon called 'phasers'. Phasers can be used by the human player to attack the computer-controlled
ships or vice-versa (see Figure 3 to see some phasers in action).
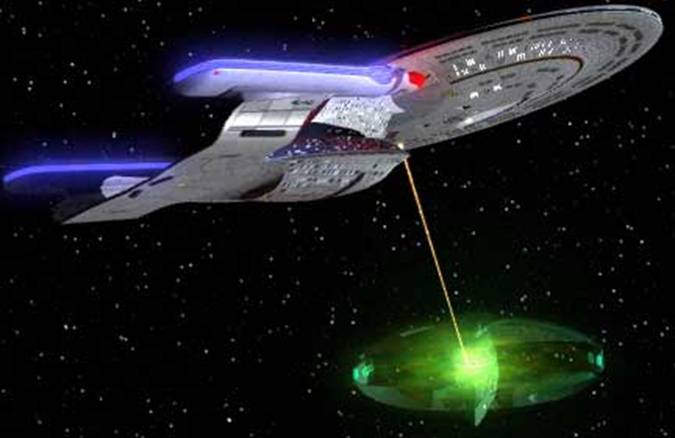 |
Figure 3: A starship
firing its phasers |
Phasers have a limited range: only the
ships in the adjacent sectors can be targeted. In the example shown in
Figure 1 the ships are too far away to shoot at each other.
In Figure 4, the ship controlled by the human player at (1, 0) and the
computer-controlled ship at (2, 0) are the only ships that can target each
other with phasers. Computer-controlled ships will only target
the ship controlled by the human player and never each other (or itself). The ship
controlled by the human player can never target itself.
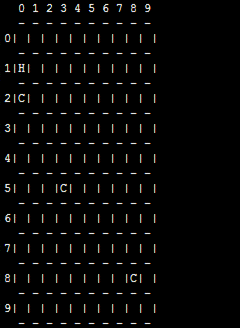 |
Figure 4: Phasers
have a limited range. |
For defense starships are equipped with a
energy shield, or 'shields' for short, that protects it from phaser
hits (see Figure 5). A ship's shields can absorb a great deal of
attacks before the energy in the shields dissipate, when the shields 'fall' or
"go down". After that phaser hits will directly damage the ship's
hull. The hull of a ship can only absorb a limited amount of damage
before it's destroyed.
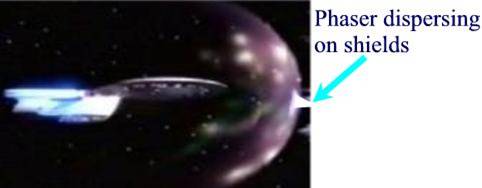 |
Figure 5: A direct phaser hit on the shields
Captain! |
Units for the phasers, shield and hull
strength are measured in terms of 'points'. The number of points
available for each of the ship's systems in this version of the Trek game
will be as follows:
- Hull: May absorb up to 400 points of damage before the ship is
destroyed.
- Shields: The number of energy points that the shields can absorb is
equal to ten times the hull points of the ship (4000).
- Phasers: Each time that they are fired they will inflict between 80
and 800 points of damage on the enemy ship.
Phaser damage is first deducted from the shields
(until they reach a value of zero) and then from the hull.
When the hull value of a ship reaches zero (or less) the ship is destroyed and it's
removed from the galaxy (that sector becomes empty).
To help illustrate how combat in this works let's use the following
example: we've played the game for period of time and we're faced
with the following situation shown in Figure 6.
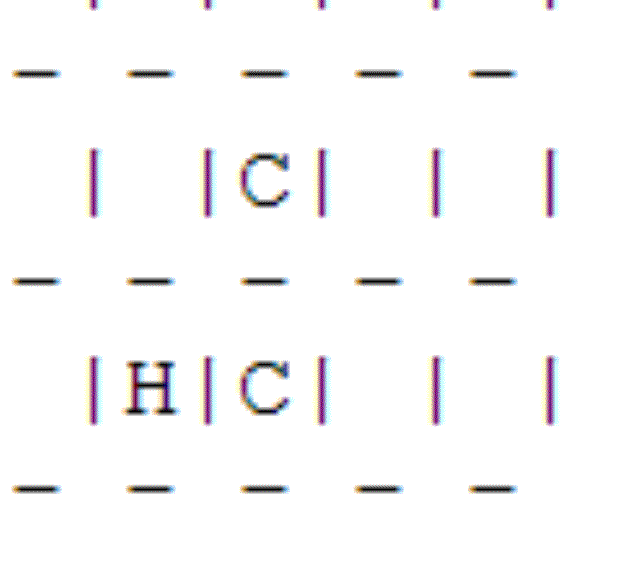 |
Figure 6 |
At the beginning of turn we have the following statistics for the 3
starships:
Human player |
Defensive system |
Points |
Shields |
925 |
Hull |
400 |
|
Computer ship
#1 (above right) |
Defensive system |
Points |
Shields |
3236 |
Hull |
400 |
|
|
Computer ship
#2 (right) |
Defensive system |
Points |
Shields |
12 |
Hull |
400 |
|
|
The human's starship targets the
starship to its immediate right (Computer ship #2) and inflicts 792
points of damage. The damage is deducted first from that ship's
shields leaving 780 points. The shield points for Computer ship #2
is now at zero (its shields are down). Since the remaining 780
points of phaser damage exceeds the 400 hull points for Computer ship #2 that
ship has been destroyed. ("Got him sir!")
However because attacks occur
simultaneously while the phaser beams for the human player's ship are
hitting the computer player's ships, the computer controlled ships are
also shooting back. Suppose that Computer ship #1 phasers
generates a mere 107 points of damage while Computer ship #2 hits back
at 880 points for a total of a whopping 987 points. Again the
phaser damage is deducted from the shields until the shields fall.
In this case the ship controlled by the human player absorb a total of
925 points of damage before failing leaving 62 points of damage that
effect the hull leaving it with 338 points. So at the end of this
round we are left with the following:
Human player |
Defensive system |
Points |
Shields |
Down |
Hull |
338 |
|
Computer ship
#1 |
Defensive system |
Points |
Shields |
3236 |
Hull |
400 |
|
|
Computer ship
#2 |
Defensive system |
Points |
Shields |
Down |
Hull |
Destroyed |
|
|
Time will pass in the game in the form of turns (when the human and
computer players can move their ships and attack). Each turn will be
broken into a number of sub-turns (some of the actions available in the
sub-turns will be implemented as extra rather than basic features):
- The computer controlled ships move.
- The galaxy is displayed.
- The human player can move his or her ship (the move menu is displayed).
Cheat options can be invoked but the use of the cheat menu will not result
in time passing (the game will still remain at this sub-turn when the cheat
menu is exited).
- The galaxy is displayed.
- The players can attack each other's ships (extra feature). Cheat
options can be invoked but again the use of the cheat menu will not result in time
passing. After this sub-turn ends the game proceeds to the next
turn (restart the list at sub-turn number one, move the computer controlled
ships).
The game will continue running on a turn-by-basis until the player quits the
game, the game has been won (extra feature) or the game has been lost (extra
feature).
Grading: Working submissions
To help you out here is a
checklist to make sure that you haven't missed
anything.
Basic submission (C+):
- Your program includes all of the
required classes (although not all the methods may be fully
implemented). See the heading "Classes for this assignment" for a
detailed description.
- Classes are instantiated and properly initialized e.g., For class
galaxy the human player always starts off in the Sol sector while the
starting positions for the computer controlled ships is randomly
determined but the appropriate array elements of the galaxy are set.
- The program displays the galaxy with each array element bound: above,
below, left and right by a numbered grid (as shown in Figure 1).
- The program displays the three menus: the movement menu, the
attack menu and the cheat menu.
Options that will be displayed by the movement menu (appears
during the movement sub-turn).
(m)ove ship (p)ass on the movement phase
(q)uit the game |
The movement feature must be implemented in the fashion described in
point #5 below. Passing on the movement phase will result in time passing
and the game proceeding to the next sub-turn. Quitting the game will
end the program with neither the win or lose game scenario occurring.
Options that will be displayed by the attack menu (appears during
the attack sub-turn):
(a)ttack an adjacent ship (p)ass on the attack phase
(q)uit the game |
The attack option is
an extra feature and is not required for the basic version of the assignment.
Passing on the attack phase will result in time passing in the game.
Options that will be displayed by the cheat menu (it can be
invoked through a hidden option from either the attack or the movement
menus):
(c)heat mode on (d)ebug mode on
(i)mpossible mode on
(e)xit the cheat menu (and return the menu that invoked it - attack
or movement). |
The ability to turn the cheat and the impossible modes on and off are not
required for the basic version of the assignment and counted as extra
features. Turning on debug mode will result in various debugging
messages being displayed (their exact content is left up to your
discretion). Exiting the cheat menu will return the player back to the
appropriate menu that the cheat menu was invoked from (attack or movement).
By itself the invocation of the cheat menu will not result in time passing
(the game will not automatically proceed to the next sub-turn when the cheat
menu has been exited).
- The human player's ship can warp to any adjacent square that is
unoccupied. The movement direction will be mapped to the numerical
keypad keys (and should be displayed as such to the player):
7 = Move up and left |
8 = Move up |
9 = Move up and right |
4 = Move left |
5 = Cancel move |
6 = Move right |
1 = Move down and left |
2 = Move down |
3 = Move down and right |
The player should not be allowed to move their ship onto an occupied
square (no ramming) nor should the ship be allowed to move outside the
bounds of the galaxy.
- The computer controlled ships randomly move to an unoccupied adjacent
square (one in nine chance of staying on the same square).
Missing an of these features will result in the loss of a letter 'step' for
each of the six listed features that are missed (down to a minimum grade of
'D-' for assignment in which a substantial amount of work was put in).
Your program must
implement the classes shown below. Note: The list of attributes and
mandatory behaviors are not meant to be as an exhaustive list (I had many
more than the ones shown below and it is likely to be the same for your
program). Also to shorten things I excluded the obvious methods that
are needed such as accessor and mutator methods as well as the constructors.
-
class Trek: This is starting point
for your program (contains the main method). Aside from declaring
a few local instances of some of the other classes and calling some of
their methods, the definition for this class should be very short.
-
Galaxy: Models all information
associated with the Galaxy.
Attributes:
- milkyWay: A 2D array of references to class StarShip. Indicates
the position of all the ships as well which parts of space are empty.
- SIZE: A final attribute to indicate how large the galaxy is (10 for
this game).
- MAX_OPPONENTS: A final attribute that indicates the maximum number
of computer players in the game (3 for this assignment).
- You will also want to track which starship is controlled by the
human player (a single instance of class StarShip) and as well as the ones that
are controlled by the computer (a list of references to class StarShip)
for easy access to the ships each turn.
Behaviors:
- Move ships from one sector to another (subject to their maximum
range which is one sector in this version of the game but it may change
in the next assignment so keep this in mind as you implement this
assignment!)
-
The program displays the galaxy along
with the bounding boxes around each sector and numbering for each row
and column.
- Checking the current state of the galaxy, determine if there are any
actions that the program has to do or what actions are available to the
players at that point in time which are dependent on the state of the
galaxy. You will likely have to implement
many methods to handle all these cases e.g., You will need a method to
check if the player is able attack any other ships this turn - you can
do this by checking if any of the adjacent squares contain a
computer-controlled ship.
- StarShip: For this game there will be four instances of
this class: 3 that are controlled by the computer and 1 that is
controlled by the human player.
Attributes:
- appearance: a character, 'H' for the human-player's ship and a 'C' for the computer's ships.
- hullValue: an integer that stores the current hull integrity (number
of points) with a starting value of 400 each time that the game runs.
- shieldValue: an integer that stores the current shield strength
(number of points). The starting value = (starting hull
value) * 10. Note: the ratio of the shield points to the hull
value may change in the next version of the game!
- current coordinates: the row/column coordinates of the ship in the
galaxy.
- maxRange: an integer value that indicates the maximum number of
sectors that a ship may move each turn. For this version of the
game it is only one but again this may change in the next assignment.
Behaviors:
- Determining the damage inflicted from the phasers when attacking
another ship for a particular turn. (Remember it must a randomly derived integer value
in the range of 80 - 800 inclusive of both end points).
- The ability to absorb the damage inflicted by other starships.
When one ship is attacked by another ship, the second ship randomly
generates a damage value for the phasers. The first ship must take
this value and determine how much the shields and hull values get
reduced. (Re-read the last section of this assignment description on
features that may added on for Assignment 5 - otherwise you may have to
totally rewrite this method when you get to the next assignment!)
- Determine the current state of the ship e.g., Is it true or false
that the shields are down? Is the ship destroyed?
- Display the current state (shield strength and hull value) of the
human player's ship after the attack phase.
- CommandProcessor: This class is responsible for getting input
from the user and for displaying the menus of options that are available
at a particular point in the game. Methods of this class
take different actions based on the user's input by invoking the methods
of the other classes (sending them messages).
Attributes:
- An instance of class Galaxy.
- The current menu option selected by the user.
Behaviors:
- Display the movement menu during the movement phase.
- Display the attack menu during the attack phase.
- Display the cheat menu as appropriate.
- Process the option selected for the movement menu.
- Process the option selected for the attack menu.
- Process the option selected for the cheat menu.
- Note: With both menus if the user selects the 'q' option then the
program will immediately end and display some sort of parting end game
message. The pass option means that the player doesn't want to do
anything during that turn (the ship doesn't move during the move phase
or the person's ship doesn't attack during the attack phase - although
any adjacent computer-controlled opponents will still attack the
player's ship). Also note that as the program ends, a check must
be performed to determine if the player won or lost the game and if
specific congratulatory or consolation message should be displayed.
In addition your program must include my GameStatus class (or you must write
an equivalent class yourself).
public class GameStatus
{
/* Required attributes */
public static boolean
debugModeOn;
public static boolean
cheatModeOn;
public static boolean impossibleModeOn;
public static boolean gameWon;
public static boolean gameLost;
}
Required attributes.
- debugModeOn: Indicates whether debugging statements show up as
the program is run. The exact content of the debugging messages is
up to you. Generally the messages that are displayed onscreen are
used to help the programmer determine where bugs are and how to fix them
e.g., when moving a starship your debugging messages may display the
current row/column coordinates as well as the coordinates of where the
ship is supposed to go.
- cheatModeOn: Cheat mode will make the player controlled starship
invulnerable. It cannot be damaged by the enemies' phasers.
Setting this attribute to true turns on cheat mode while setting it to
false turns off cheat mode.
- impossibleModeOn: Determines whether the computer controlled ships
move randomly or whether they move towards the human player's ship.
Optional attributes.
- gameWon: This attribute is set to true when the win game condition
(see above) is reached.
- gameLost: This attribute is set to true when the game is lost (see
above).
- (It is possible for the player to quit the game without either the
game win or game lost condition being met).
Of course if one these optional
attributes is true then the other cannot be true. They can be used in
different parts of your program to determine if it is time to end the game.
Extra features (implementing each feature increases your grade by one
letter step, completing all these features can yield a grade of "A+")
Unless otherwise stated the extra features can be completed in whatever
combination and order that you desire.
Feature 1: Implement the 'impossible' level of difficultly in
the cheat menu. When the game is set to this difficultly level, the
computer-controlled ships will no longer move in a random fashion but instead
will move towards the player's ship (unless another computer controlled ship is
in the way). Turning off the impossible difficulty level will result in
the computer-controlled ships going back to a random pattern of movement.
The game should indicate to the player when it is in impossible mode.
Feature 2: Implement the attack option in the attack menu so that the
human player can attack an adjacent computer controlled ship. Use the same
directional grid that you used for movement to show the player the attack
directions.
Feature 3: Computer controlled ships can attack the human player's
ship if it's adjacent.
Feature 4 (Feature 2 must be implemented first): The game will check
if the win game condition has occurred after the attack phase. If all
three computer controlled ships have been destroyed then the game will end a
suitable congratulatory message should be displayed.
Feature 5 (Feature 3 must be implemented first): The game will
check if the lose game condition has occurred after the attack phase. If
ship controlled by the human player has been destroyed then the game will end a
suitable consolation message should be displayed.
Feature 6 (Feature 3 must be implemented first): Implement the
'cheat mode' option in the cheat menu. There are two approaches that you
can take. When the cheat mode is on the computer controlled ships can either
appear to attack the player's ship with no apparent effect (it appears
invulnerable) or the computer controlled ships simply won't attack the player's
ship during the attack phase. When cheat mode is turned off any adjacent
computer controlled ships will resume their attacks. The game should
indicate to the player when it is in cheat mode.
Feature 7: Only show the attack menu when the human player's ship is adjacent to a
computer controlled ship. If this is not the case then the attack phase
and second display of the galaxy should be skipped.
Grades for non-functional assignments
D level assignment
The student has invested considerable work in the assignment1 and the code
compiles but it doesn't fulfill any of the above requirements.
D- level assignment
The student has invested considerable work in the assignment1 but it doesn't
compile.
Other submission requirements
In addition to having fulfill the
generic assignment requirements the
requirements specific to this assignment include:
-
Good coding style and documentation:
They will play a role in determining your final
grade for this assignment. Your grade can be reduced by a letter step or more
(e.g., 'A' to 'A-' for poor programming style such as employing poor naming
conventions for identifiers, insufficient documentation or the use of static
variables or methods). For additional details see the
marking guide for coding style.
-
Include a README file in your submission: For
this assignment your README file must indicate what grade level has been
completed (e.g., 'A') and which features have been implemented This will allow your
marker to quickly determine what he or she must look for and speed up the
marking process. Also you should
include your contact information:
your name, university identification number and UNIX
login name so that your marker knows whose assignment that he or she is
marking. Omitting the necessary information from this file will result in the
loss of a letter 'step' (assuming that the marker can actually figure out who
the assignment belongs to, if it cannot be determined
who the assignment belongs to then no grade will be given for the assignment,
an 'F' will be assigned).
-
Assignments (source code/'dot-java' files and the README file) must be
electronically submitted via email (to
me and your TA).
In addition a paper print out of the source
code and README must be handed into the assignment drop box (located on the
second floor of the Math Sciences building) for the tutorial that you are
registered in. Electronically submitting the assignment allows your marker to
run the code in order to quickly determine what features were implemented.
Providing a paper printout makes it easier for your marker to read and flip
through your source code. Omitting the paper version of the source code file
will result in the loss of a letter 'step'. Omitting the electronic version
of your assignment will only allow you to receive a maximum grade of
'D-' because it's too time consuming for your marker to check every
program with a hand trace. I suggest that as you complete the various
features of the assignment that you immediately submit each version so if
you forget to submit the final version you will at least have something that
your marker can grade because you won't be allowed to submit anything after
the deadline.
-
As a reminder, you are not allowed to work in groups for this class.
Copying the work of another student will be
regarded as academic misconduct (cheating). For additional details about what
is and is not okay for this class please refer to the following
link.
Relevant Files
You can run the sample byte code file called
'Trek.class' which can be found in Unix in the directory:
/home/233/assignments/assignment4
1 |
What does and doesn't constitute a sufficient amount of time and
effort? It's a judgment call on the part of your marker. More often
than not if you put in a reasonable amount of effort into your assignment and
for some reason you just couldn't get it to work then you will receive some credit
for your work. An example of when you wouldn't receive credit is
when you simply handed someone else's work. This latter case assumes that
you properly cited the other person's work, if you didn't cite your source and tried to claim
that it was your own work then it would be an example of academic misconduct
(cheating). |
Future add-on that may apply for Assignment 5:
These are some things to keep in mind as you design this program because if
you make your program too specific for this assignment without considering
possible future extensions then you may have to rewrite major sections of your
Assignment 4 code.
- Travel is possible at speeds faster than warp (thus extending their
movement range each turn).
- Cloaking devices have been invented (the invisibility allows opponents to
attack before the defender can react).
- Opponents can adapt their shields to your weapon attacks (so your phasers
do less and less damage to them over time).
- Weapons have been developed that can penetrate shields (and directly
damage a ship's hull).
- Ships are equipped with regenerative shielding (each turn the shields will
increase in points if they are below their maximum value).
Note: The use of the Star Trek © trademark was
for educational purposes only and not meant as a copy write challenge.