A3: VBA Programming For Word
Due dates for all assignments can be found on the [graded
components]
section of the course webpage. Most students will find this assignment more
challenging than any of the previous assignments so you should start working on
it as soon as possible. Don't wait until all the VBA lectures are finished, once
we're covered any material related to the assignment you should immediately
implement the appropriate part of the assignment. You won't be granted extra
time if you find the assignment more challenging than you expected.
Here is a [starting document]
to ensure that you at least start with the type of Word document that can
contain a macro (i.e. a "Macro enabled Word document). (It doesn't contain any
VBA instructions as you need to do this yourself). The document is provided
merely to get you started, whether or not you use it is entirely up to you. If
you are unsure of what is required in the creation of a VBA program then you
should refer to your in class lecture notes (the
first set of VBA lecture notes, VBA Part I) but here is a reminder image of
how you should save your macros:
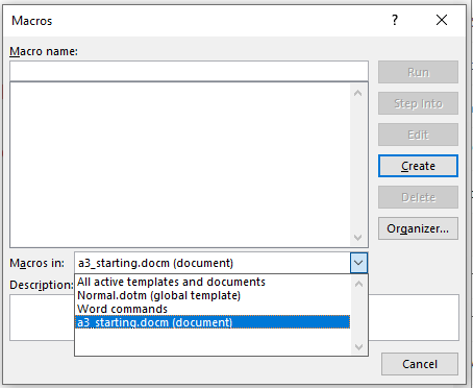
Because your macros will be included in the document, when it comes time to
submit your work you can just upload the macro-enabled document. Double
check that your VBA macro really is in the Word document that you upload! It
is up to you to do the check, you shouldn't rely on us to do it for you. If you
want to be extra safe (and I highly recommend that you do this) you can copy and
paste your VBA program into a regular Word document and submit that extra Word
document as well the macro-enabled document. Or
if you copy paste the program into Word document that contains your macro then
you don't need to submit an additional document.
As was the case with other assignments do not use other compression utilities
(such as zip), otherwise your submission may not be marked.
Caution and tip for the assignment
Similar to the previous workbook exercise this assignment will be challenging.
In fact it will be significantly more challenging and take more time than the
exercise. So you should start working on it as soon as possible. You won't be
given extra time if you find yourself "in over your head" and you cannot
complete the work before the deadline. On the other hand previous students have
been able to complete a good portion of the requirements in previous semesters.
Most of them did not have any programming experience so this assignment is one
that beginners do have the ability to complete if they apply themselves properly
and if they are prepared to put in the time. (Reminder: it involves more than
just trying to write the code for this assignment and the exercises, you should
try to practice as much as possible beforehand: writing programs and tracing
programs in order to develop your skills at both).
Write a VBA macro that includes the following features
(note that failing to follow style requirements can
affect your grade)
Implementing Features #1 - 5 without implementing the last
feature will have these features affect the currently active Word document.
Implementing Feature #6 will have Features 1 - 5 apply to all the Word
documents in a location specified by the user.
- Prompt the user for a word using an InputBox.
(0.1 GPA)
If you implement Feature #6 this word will be the same search Word that the
program will try to find in each document at the specified location.
- Count the number of instances of the word (from
Feature #1) using the appropriate parts of the ActiveDocument object.
The count should include partial matches e.g. if the input word was 'jam'
then 'James' would
be counted as match. As you can see in the example, the counting process is
not case sensitive. If Feature #6 is implemented (multiple documents are
searched) then the same search word should be used for all documents.
You will only be awarded 0.2 out of 0.4 GPA if your program requires new
prompt for each document opened. (0.4 GPA)
- Display the count of the instances via a MsgBox
in the following form. "Number occurrences: "<actual number of occurrences of the search word> e.g. Number
occurrences: 2 (0.1
GPA)
-
Write information about the number of instances into the currently active
Word document using the appropriate parts of the Selection object.
(If Feature #6 is implemented then this information would be written into
the respective document in which the count was being conducted). Example of
a document (counting number of instances of 'silent') [before
program counts] [after count]. Note:
Features 4d & 4e only affect the text that your program writes to the
document, the formatting of the rest of the text in the document is
unchanged.
- The text written should take
the form: "Number occurrences:
<actual number of occurrences of the search word>" e.g. Number
occurrences: 2 (0.3
GPA)
- This text should appear on its own
line i.e. it should be preceded by "a hard return" or a VBA carriage
return "vbCr".
(0.3 GPA)
- The text appears at the end of the
document ("end of story"). (0.3 GPA)
- If the search word (entered with
Feature #1) appears two times or more then the text (from Feature #4a) to be written
(Feature 4a) is colored red. Credit will be awarded if the text is
colored under the correct condition. (0.4 GPA)
- If the search word appears three or
more times then the text to be written (from Feature #4a) is also bolded.
Credit will be awarded if the text is bolded under the correct
condition. (0.4
GPA)
-
Increase the 'accessibility' of the document: If there are any images in the
document then they will be modified using the appropriate parts of the ActiveDocument object.
- Double the size (both the
height and width change so the proportions of height to size remains the
same) of each image (in VBA "InLineShapes"
in the document. Keep in mind that some methods will automatically
retain the proportion when just one of the dimensions is changed. (0.3 GPA)
- If the document doesn't contain any
images then a MsgBox will
appear with the message "No images to
modify". (0.2
GPA)
-
Modify multiple documents (automating a repetitive task): Program runs as
above but it will run Features 2 - 5 on all the Word documents at a
location specified by the user. That is, the above 4 features (2-5) are
contained in the body of a loop defined for Feature #6. Again: Feature #1
runs only once, the program searches for the same word for all Word
documents.
- Prompt the user for the
location of the Word documents using an InputBox e.g.
e.g. "C:\203".
The program should not require that the user enters a slash after the
name of the last folder (in this example the containing folder is '203')
because your VBA program will automatically add it to the end before
trying to open any documents. (0.1 GPA)
- If the user enters an empty location
i.e. presses "OK" without specifying a path then
the program will display a MsgBox that
contains the message "No location entered,
ending program" and then the program will
end. Hint: you don't need to use a special instruction to end the
program. Reaching the end of the subroutine (or part of the subroutine)
will automatically end the program. (0.2
GPA)
- If the location is not empty then the
program will successively open each Word document the at that location
using the appropriate parts of the
Documents collection. To get credit for
this feature and the next feature the program must not only open just
Word documents but only open certain types of Word documents: older
Word 97-2003 (.doc)
documents, newer Word 2007+ (.docx)
documents and even macro enabled documents (.docm).
If the folder contains any other type then your program must be able to
'ignore' them (not open them) and only open these 3 types of Word
documents. (0.2 out of 0.5 GPA if the program tries to open all files in
the specified location even if they aren't Word documents). (0.5
GPA)
- After opening each Word document the
program will apply Features 2 - 5 on each document. (0.5
GPA)
Documentation requirements
-
Contact information: your full name as provided to the university (make sure
it matches, don't include 'nicknames'), student identification number,
tutorial number. (0.045
GPA)
-
Specifying clearly what features of the assignment that you completed or
didn't complete. In order to get credit the documentation has to be clear, complete
and of course correct. [Example] (0.155
GPA)
- Each level of code indenting is
consistently 1 tab. Instructions
in the sub-routine (between the 'sub'
and 'end-sub' is 1
level, the body of IF or WHILE structures
counts as another level of indenting. An IF within
an IF or WHILE within
a WHILE (or even
an IF within a WHILE or
a WHILE within an IF) each
count as another level. Penalty: -0.2 GPA applied
to any case (and not each case) where the required indenting is not applied
and applied consistently.
- Good naming conventions (e.g. variables,
sub-routines, the name of Word document containing the VBA program and
constants if applicable) are followed. Some examples of naming conventions
are provided in [the VBA Part I
notes]. Penalty: -0.2 GPA applied
to any case (and not each case) where poor naming conventions have been
used.
JT's cautions: these are
oddities that I encountered when I was making up my solution.
Number 1: If
your images get too large (i.e. there's a maximum size allowed) then when
you run your program and it tries to enlarge an image you will get a
"runtime error".
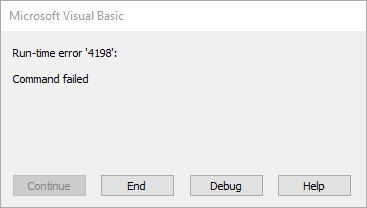
If then select 'Debug' you may see VBA flag the
instruction that tries to enlarge an image as the source of the problem.
Number 2: Order is
important. When writing text to the Word document or documents (Feature #4)
put the instruction that tells VBA to write the text to the end of the
document before any instructions that format the text (bold and red
font). Reversing the order may result in no formatting effects for the font
even if the number of instances of the search Word is sufficiently high. Look at this [example]
that I created which illustrates the effect changing the order. The text
inserted at the end is not colored red when the person writing the program
wanted it be colored. On the other hand the text inserted at the start of
the document is colored red. Note the difference in the ordering of the
statement between the two insertions.
-
Operating system specific issues: submissions
must work on the machines a Windows computers. (For the remote learning
semester since access to the lab computers is more challenging: the
requirement is that your document works on any Windows computer). It's up to
you to test and check this is the case. Non-functional submissions will
receive only partial credit (if any at all). Although
operating system issues are rare (non-existent?) if you implement your
solution on another operating system it's a good idea to check your
submission on a Windows computer. (See the administrative video lecture
loaded into D2L for the week of
Jan. 9 - 15 for suggestions as to how you can do this). Caution for
Windows users: take care that you don't
accidentally submit a shortcut to a file instead of the actual file.
(Check the file name and compare the file size to your original file. Simply
downloading the shortcut file as a test won't work because that shortcut
will work on your computer but not on anyone else's machine).
-
Late assignments or
components of assignments: Extensions may
be granted for reasonable cases by the course instructor with the receipt of
the appropriate documentation (e.g., a
statutory declaration with a commissioner of oaths). Location of the
online form: [PDF
file]. Alternate submission mechanisms (non exhaustive list of examples:
email, uploads to cloud-based systems such as Google drive, time-stamps, TA
memories) cannot be used as alternatives if you have forgotten to submit
work or otherwise have not properly submitted into D2L. Only files
submitted into D2L by the due date is what will be marked,
everything else will be awarded no credit. The final cut off date after
which full assignments will not be accepted is after the maximum
progressive penalty (listed below) can be applied.
-
Assignment extensions (must
be requested before the assignment is due): may be granted for reasonable
cases by the course instructor with the receipt of the appropriate
documentation (e.g., a
sworn declaration signed by a commissioner of oaths). Typical examples
of reasonable cases for an extension include: illness or a death in the
family. Example cases where extensions will not be granted include
situations that are typical of student life: having multiple due dates, work
commitments, forgetting or mixing up due date etc. Tutorial instructors
(TAs) will not be able to provide extension on their own and must receive
permission from the course instructor first. If
you request an extension from me let me know the name of your tutorial
instructor and the tutorial number [link to : tutorial
and TA information) because the markers won't accept
late submissions without directly getting an email from me and I need to
know who to contact. Also extensions need to be requested before the
due date and not afterward.
-
How you will be graded for assignments.
Feedback will be provided by the Teaching assistant in a [marking
checklist]. Besides seeing your grade point in D2L you can also see the
detailed feedback in this spreadsheet that your TA will enter for each
student because it will uploaded for each assignment into the D2L Dropbox.
You can access the grading sheet in D2L under Assessments->Dropbox and
then clicking on the appropriate assignment link. If you still cannot find
the grading sheet then here is a [help
link]
-
Questions or
concerns about grades have been released: Assignments will be marked by
your tutorial instructor (the "Teaching Assistant" or "TA") for your tutorial
section (instructor information listed here as well). When you have
questions about marking this is the first person that you should be
directing your questions towards. If you still have question after you have
talked to your TA, then you can talk to your course
(lecture) instructor but please indicate in your email that you first
contacted your TA before going into your concerns.
Collaboration:
Assignments must reflect
individual work;
group work is not allowed in this class nor can you copy the work of others.
Some "do nots" for your solution: don't publically
post it, don't email it out, don't show it to other students,
don't get help with your assignment from a tutor (if you have hired one).
For more detailed information as to what constitutes academic misconduct (i.e.,
cheating) for this course please read the following [link].
Submitting your work:
-
The document must be electronically submitted using D2L.
-
Submit a macro-enabled document (docm)
containing your program which should be accessible via the Visual Basic
editor. As a precaution it's recommended that you copy-paste the VBA program
into the body of the Word document that you are submitting in case you
didn't properly save your program in the docm
document.
-
Make sure that you [check
the contents of your submitted files]
(e.g., is the file okay or was it corrupted, is it the correct version
etc.). It's your responsibility to do this! (Make sure that you
submit your assignment with enough time before it comes due for you to do a
check). If don't check and there were problems with the submission then you
should not expect that you can "learn your lesson" and simply resubmit.
-
Do not use compression utilities (such as zip) or archiving utilities
(such as tar) otherwise your submission may not be marked. The space savings
in D2L is not worth the extra time required by the marker to process each
submission.
-
How often can you submit: Multiple
submissions are allowed for this assignment: You can (and really should)
submit work as many times as you wish before the due date. Due dates are
strict, only what is in D2L by the deadline is what will be marked. Other
methods of verifying that your work was completed on time (e.g. checking
timestamps, emailed files etc.) will NOT be accepted.
However only the latest versions of each individual document are the ones
that will be marked, everything else will be ignored (because it is not fair
to your marker to sort through multiple versions of your files). In the case
of A1 the marker will just look at the latest version of: Calgary and the
merged letter that the marker can 'click
through'.
Late
submissions for assignments when there is no extension granted: Make
sure you give yourself enough time to complete the submission process so
you don't get cut off by D2L's deadline (or your submission will be
automatically flagged as late by D2L and it will be graded appropriately)..
Submission received: |
On time |
Hours late : >0 and <=24 |
Hours
late: >24
and <=48 |
Hours
late: >48
and <=72 |
Hours
late: >72
and <=96 |
Hours
late: >96 |
Penalty: |
None |
-1 GPA |
-2 GPA |
-3 GPA |
-4 GPA |
No credit
(not accepted) |