CPSC 231: Assignment 4
New Concepts to be applied for the assignment
- Lists
- Implementing a solution for a moderately complex problem
- Employing debugging tools
Introduction
Love or hate it Star Wars1 has had an undeniable
impact on the movie industry by creating the tradition of the "summer block
buster". The movie begins with a rebel ship carrying the plans for the
"Death Star" (a moon sized mobile base ship capable of destroying whole planets) being
boarded by the infamous Darth Vader. Through a complicated series of events
the young farm boy, Luke Skywalker, becomes involved in the plan to destroy
the Death Star. The movie culminates with Luke making a desperate strafing
run at the only vulnerable location on the Death Star (the exhaust vent)
just as it is coming into range of the rebel base.
For those who want to read more Star Wars trivia you can go to the following
link on the IMBD. The information used to create this introduction comes largely
from the Internet Movie Database (http://www.imdb.com/title/tt0076759/). |
|
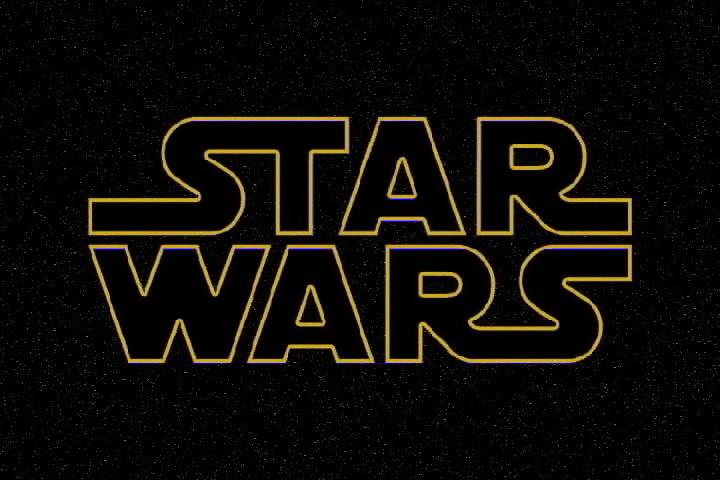 |
Assignment description
For this assignment you are to implement a
text-based semi-side scrolling Star Wars arcade game. This game will re-create the scene where Luke
makes a strafing run in the equatorial trench (shown in Figure 1) of the Death
Star's (shown in Figure 2) one vulnerable location, the exhaust vent. If he can
score a direct hit then the explosion will set off a chain reaction that will
destroy the entire base (player wins the game). However there is only time
to make one attack run so
if he is destroyed or if he misses/over flies the vent then all is lost because
the Death Star will destroy the rebel base (player loses the game). The
situation is made even more desperate as Luke must brave the gauntlet of enemy tie-fighters.
The game will
continue running until: the player has won the game, the player loses the game
or the player quits the game.
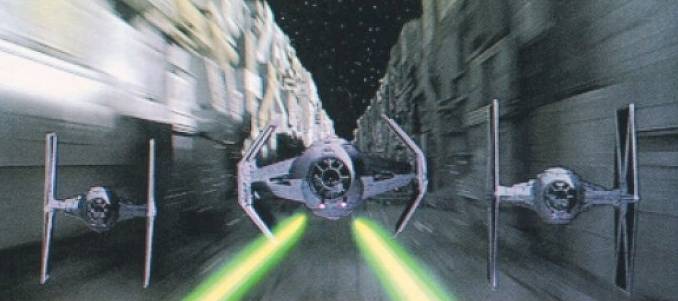 |
Figure 1:
The equatorial trench,
the scene of your game! |
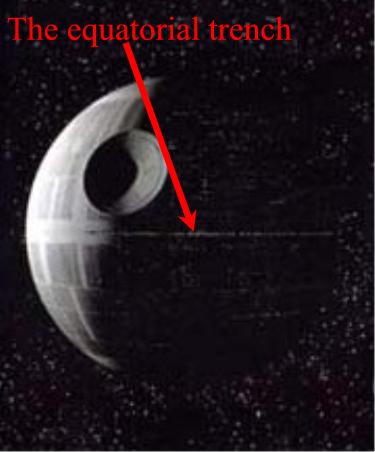 |
Figure 2:
The Death Star ("That's no moon!"). |
To win the game the player must successfully
fly Luke's X-wing fighter (represented aptly enough by the upper case 'X'
character) in a strafing attack run on the exhaust vent (represented by the
'v'). Solid walls are represented by the '*' character, open areas by the space
character and the tie fighter by the upper case 'H' (JT: don't the tie
fighters in Star Wars look like flying H's to you?) The player loses the
game if either Luke's ship has been destroyed (by crashing into the tie fighter
or the walls) or if he misses the vent (the ship reaches the 20th column (column
J at the top right of Figure 3).
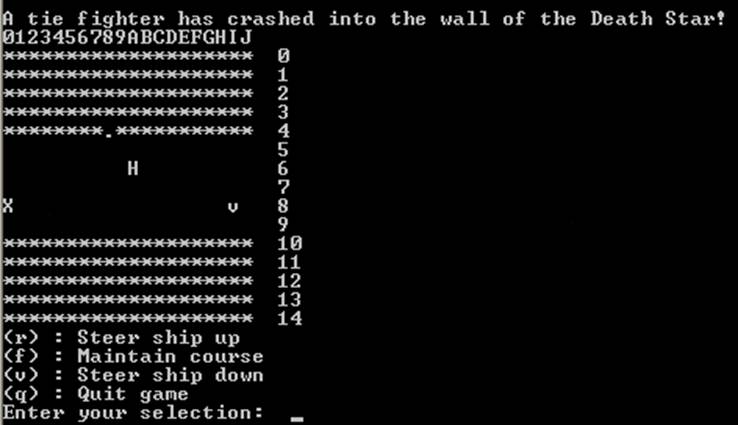 |
Figure 3: Screenshot of the game |
The Death star will be represented by a 15x20 two-dimensional list (rows
5 - 9 in Figure 3 show the trench). The game starts out by initializing the
list to the default starting character values and displaying onscreen a brief
introductory message about the game. After that the game will run on a turn
based fashion until the user decides to quit (i.e., selects 'q' at the main
menu) or if the lose or win game conditions have been met. Each turn will be
divided into a number of sub-turns:
- Move the tie fighter.
- Display the trench.
- Display the main menu.
- Move Luke's ship.
- Check the game status, determine if the player has won or lost the game
and set the appropriate flag.
- If the player has won or lost the game then an appropriate concluding
message will be displayed along with the final state of the trench (character
positions) and the
program will end. If the player opts to quit the game early, then neither the
win game nor lose game message will appear and the program will simply end.
If the player doesn't choose to quit the game and if the game hasn't been won
or lost, then the program will return to step #1 until one of the end game
conditions has been met.
Features to implement:
Note: you must use the program in the UNIX directory:
/home/231/assignments/assignment4 for determining the starting positions of the
all objects in the game.
- Displaying the Death Star with numbered annotations along the rows and
columns.
- Show the main menu with the options for controlling Luke's X-wing fighter
and getting the player's selection (see Figure 3). There will be options for
moving Luke's ship and one option for exiting the game (which ends the game
which is neither won nor lost by the player).
- Moving Luke's ship. The player can either move the fighter up or down. In
either case the ship will also move forward one column. Figure 4 shows Luke's
fighter moving from (8,0) to (7,1). The player moved the ship up but the
fighter automatically moved forward as well. (Regardless of the direction that
the player chooses to move the ship it will always move forward one square). The player also the option of not
maneuvering the ship in which case it will just move forward by one. If
Luke's ship moves into the walls of the trench or into a tie-fighter then the ship
is destroyed and the player loses the game (which then ends with a suitable
status message). The location of the destroyed ship should be marked with an 'X'. If Luke's
ship flies onto the trench then he's successfully launched his torpedo
destroying the Death Star and the player wins the game (which then ends with
another suitable status message). If Luke's X-wing fighter reaches column J
then he has over flown the vent and because he only has time to make only one
pass through the trench (before the Death Star fires on the rebel base) then
the player loses the game (which should again end with another different suitable
lose game message).
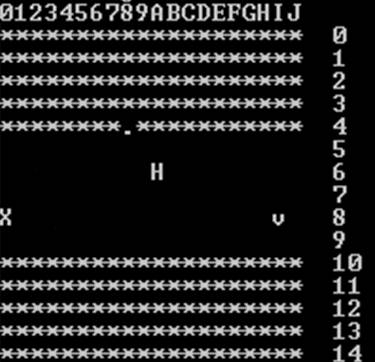 |
|
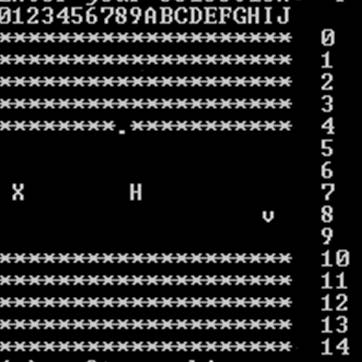 |
Figure 4A: Luke's fighter before
the move |
|
Figure 4B: Luke's fighter after the
move |
- The game has a single tie-fighter that randomly moves to an adjacent
square each turn. In this case adjacent refers to squares that immediately
surround or touch the square where it currently resides. (Refer to Figure 5).
Tie-fighters won't move onto the exhaust vent but they can accidentally fly
into walls - as shown in Figure 4- (or each other - if your version of the game includes multiple
enemy opponents). If a tie-fighter crashes into the wall it should leave
behind some debris (marked by the period '.'). (For the version of the game
with multiple opponents if another tie-fighter flies into the same part of the
wall the period should be cleared (become an empty space). If Luke and a
tie-fighter happen to hit the same spot on the wall it will simply be marked
by an 'X' after Luke's collision.
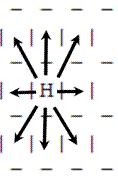 |
Figure 5: A tie fighter can move to
any adjacent square. |
- The game includes a hidden 'cheat' feature. Although the main menu only
shows options for moving Luke's ship or quitting the game if the player enters
'c' then a hidden cheat menu will appear. (Figure 7)
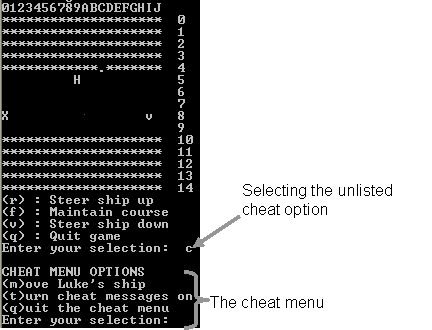 |
Figure 6: Invoking the cheat menu
through a hidden option at the main menu. |
The 'move' option will then show prompts to allow the player to enter the
row and column that they wish to move Luke's fighter to. Entering a negative
row or column value is the equivalent of selecting the quit option at the cheat menu and will
bring the player back to the main menu. This feature is especially
useful for testing your program e.g., quickly seeing if collisions are handled
properly or if the win game condition is working. Note: if the player entered
a negative value when prompted to enter the row value then the program should
not prompt the person to enter in a column value but instead the game should
just proceed back to the main menu.
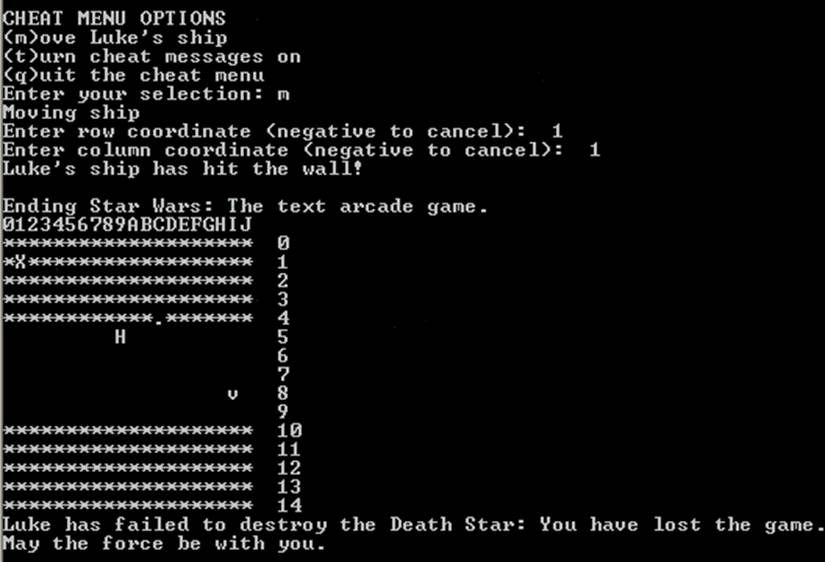 |
Figure 7: The move option in the
cheat menu allows the player to move Luke's ship onto any square in Death
Star. |
Turning cheat messages on will display debugging messages for your program.
Selecting this option again will turn the debugging messages off. The exact
content of the messages are up to you but they should be used to help you test and
debug your program. Some suggestions: showing the name of a function as it's
called, displaying the previous (row/column) coordinates of ships and the
coordinates of the ships after they have moved.
- The final feature of the game involves getting a version that
works with multiple (2) tie-fighters. It should implement the above features
as well as handle the new case: a tie-fighter to tie-fighter collision. (JT's
hint: since this feature is harder than all of the others I suggest that you
work on it only at the very end).
Submitting your work:
- Assignments (source code/'dot-py' files containing your Python program and your README) must be electronically submitted
according to [the assignment submission
requirements].
- As a reminder, you are not allowed to work in groups for this class.
Copying the work of another student will be
regarded as academic misconduct (cheating). For additional details about what
is and is not okay for this class please refer to the following [link].
- Before you submit your assignment to help make sure that you haven't
missed anything here is a [checklist] of items to be used in marking.
Videos showing examples of program execution:
- Luke's ship flying up and down, tie-fighters randomly moving: [cruising]
- Using the cheat menu to win the game: [cheat]
- Showing cheat messages (debugging statements): [debug]
- Crashing into the wall of the DeathStar with the lose game result: [crashed]
- Over flown the trench and losing the game: [over
flown]
- Winning the game by hitting the exhaust vent: [win]
1 Star Wars is ©
Lucasfilm Ltd. References to either the this trademark or the inclusion of
images from the movies are for education fair use only and are not meant as a
copy write challenge.