CPSC 219: Assignment 4
New Concepts to be applied for the assignment
- Solving a moderately sized problem using the Object-Oriented approach
- Writing programs with multiple classes
- Relationships between classes
- Message passing
Introduction
It's hard to
believe but the "Trek" franchise has been around for over 40 years.
Production of the first episode "The Cage" began in late 1964. It was
given a budget by NBC of $640,000 and produced by Desilu Studios (which
was since taken over by Paramount Studios which has now become part of the
media giant Viacom). Since that historic date, Star Trek has become
a part of television and motion picture history and includes an seven
separate TV series and eleven motion pictures. |
|
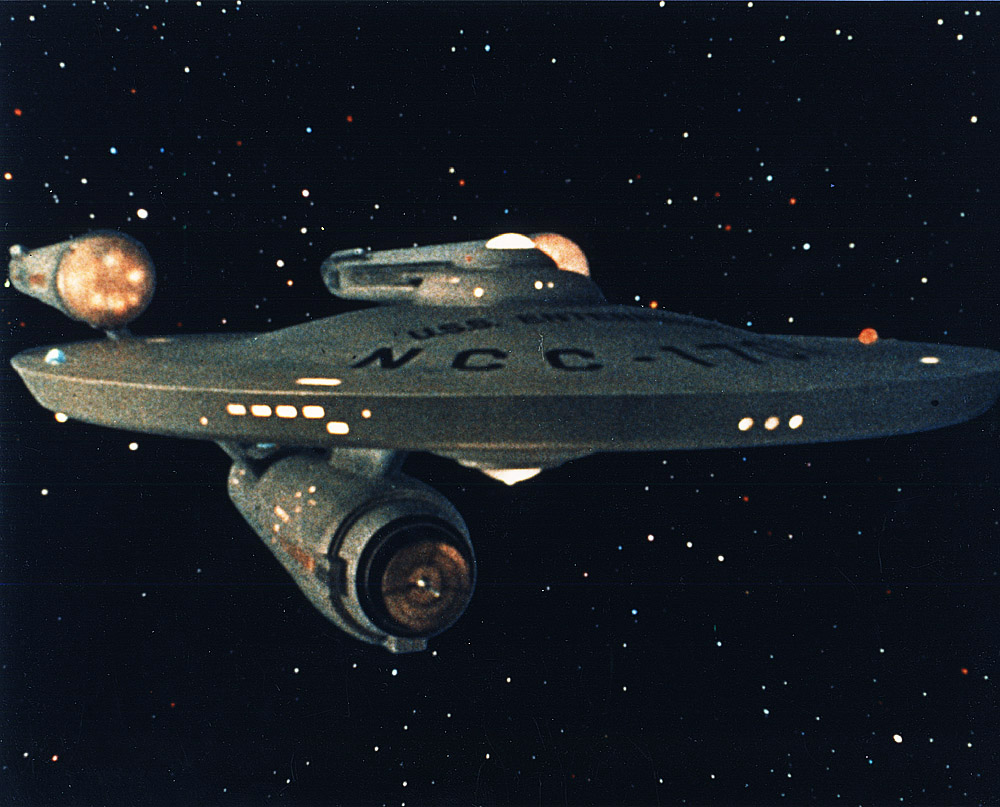 |
Your mission for this assignment
For this assignment you are to implement a
simple Star Trek game. The Trek galaxy (i.e., the milky way) will be modeled
with a ten-by-ten two-dimensional array of references. Each array element
(called a "sector") will either be null (representing empty space) or contain
a reference to a starship. Starships will be represented either by the 'H'
character (which is the ship that is controlled by the human player) or a 'C'
(for the three computer-controlled ships). When the game starts, the human
player's starship will always start off in the same location, the "Terran
sector", coordinates (0,0). The coordinates of the three
computer-controlled ships will be randomly determined (see Figure 1 for an
example of the initial starting configuration of the Galaxy). To make it
easier to make sense of where things are located, the galaxy is bounded by a
numbered grid. above, below, left and right of each array element.
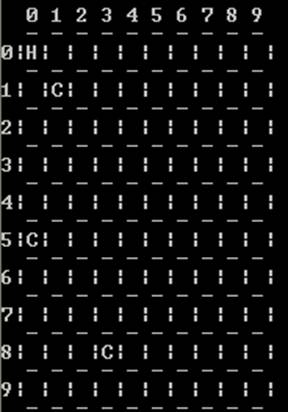
Figure 1:
Example starting positions.
|
Starships are equipped with
faster-than-light engines known as "warp drive". In terms of the game a ship
can move into one of the adjacent sectors during the move phase (see Figure
2). Even with warp engines a ship still cannot leave the galaxy (i.e., exceed the bounds of the array).
 |
Figure 2: Warp drive
can take a ship to any of the adjacent sectors |
Each starship equipped with a powerful (~1
Gigawatt) energy beam weapon called "phasers" (NOT to be confused
with puny lasers). Phasers can be used by the
human player to attack the computer-controlled ships or vice-versa (see Figure
3 to see some phasers in action).
 |
Figure 3: A starship
firing its phasers |
Phasers have a limited range: only the
ships in the adjacent sectors can be targeted. In the example shown in Figure
1 the human player's ship can only shoot at one computerized opponent (and
vice versa). In Figure 4, the
ship controlled by the human player at (1, 1) and the computer-controlled ship
at (2, 1) are the only ships that can target each other with phasers. Note:
Computer-controlled ships will only target the ship controlled by the human
player and never each other. The ship controlled by the human player can
never target itself.
 |
Figure 4: Phasers have
a limited range. |
For defense starships are equipped with a
energy shield, or "shields" for short, that protects it from damage (see
Figure 5). A ship's shields can absorb a great deal of attacks before the
energy in the shields dissipates, the shields "fall" or go "down". After that
phaser hits will directly damage the ship's hull. The hull of a ship can only
absorb a limited amount of damage before the ship is destroyed.
 |
Figure 5: A direct
phaser hit on the forward shields! |
Units for the phasers, shield and hull
strength are measured in terms of "points". The number of points available
for each of the ship's systems in this version of the Trek game will be as
follows:
- Hull: May absorb up to 400 points of damage before the ship is
destroyed.
- Shields: The number of energy points that the shields can absorb is
equal to ten times the hull points of the ship (4000).
- Phasers: Each time that they are fired they will inflict between 80 and
800 points of damage on the enemy ship.
Phaser damage is deducted from the opposing ship first from the shields
(until they reach a value of zero) and then from the hull. When the hull
value of a ship reaches zero the ship is destroyed and is removed from the
galaxy (that sector becomes empty). If the player's ship is destroyed then the
game is lost and it ends with an appropriate status message displayed. If all
three computer controlled ships are destroyed then the game is won and the
game should end with another status message.
Time passes in the game in terms of "turns". During each turn the
following sub-turns will take place.
- The computer player moves its three ships
- The human player is given a chance to move his or her starship. The
player is allowed to "pass" and not move the ship.
- The current state of the galaxy is displayed.
- The player's starship and any computer-controlled starships that are
adjacent to the player's starship attack each other. In this version of the
game attacks occur simultaneously (i.e., during a turn a computer-controlled
starship and the player's starship could end up destroying each other at the
same time). Again the player can pass on the attack phase although his or
her ship will still be attacked by its computer controlled enemies.
- At the end of the turn the program checks if the "game win" or "game lost"
conditions apply. The player wins the game by destroying all of the
computer's starships. The player loses the game when his or her ship is
destroyed. It's also possible that the game ends but is neither won nor lost
by the player (i.e., the person quit the game prematurely).
To help illustrate how combat in this works let's use the following
example: we have played the game for period of time and we are faced with
the following situation shown in Figure 6.
 |
Figure 6: Example
combat |
At the beginning of turn we have the following statistics for the 3
starships:
Human player |
Ship's system |
Points |
Shields |
925 |
Hull |
400 |
|
Computer ship #1 |
Ship's system |
Points |
Shields |
3236 |
Hull |
400 |
|
|
Computer ship #2 |
Ship's system |
Points |
Shields |
12 |
Hull |
400 |
|
|
The human's starship targets the starship
to its immediate right (Computer ship #2) and inflicts 792 points of
damage. The damage is deducted first from that ship's shields leaving 780
points. The shield points for Computer ship #2 is now at zero (its shields
are down). Since the remaining 780 points of phaser damage exceeds the 400
points for Computer ship #2 that ship has been destroyed. ("Got him sir!")
However because attacks occur
simultaneously while the phaser beams for the human player's ship are
hitting the computer player's ships, the computer controlled ships are also
shooting back. Suppose that Computer ship #1 phasers generates a mere 107
points of damage while Computer ship #2 hits back at 880 points for a total
of a whopping 987 points. Again the phaser damage is deducted from the
shields until the shields fall. In this case the ship controlled by the
human player absorb a total of 925 points of damage before failing leaving
62 points of damage that effects the hull leaving it with 338 points. So at
the end of this round we are left with the following:
Human player |
Ship's system |
Points |
Shields |
Down |
Hull |
338 |
|
|
Computer ship #1 |
Ship's system |
Points |
Shields |
3236 |
Hull |
400 |
|
|
Computer ship #2 |
Ship's system |
Points |
Shields |
Down |
Hull |
Destroyed |
|
|
Classes for this assignment
Your program must
implement the classes shown below. Note: The list of attributes and mandatory
behaviors are not meant to be as an exhaustive list (I had many more than the
ones shown below and it is likely to be the same for your program). Also to
shorten things I excluded the obvious methods that are needed such as accessor
and mutator methods as well as the constructors.
-
Trek: This is starting point for
your program (contains the main method). Aside from declaring a few local
instances of some of the other classes and calling some of their methods,
the definition for this class should be very short.
-
Galaxy: Models all information
associated with the game universe.
Attributes:
- grid: A 2D array of references to class
StarShip. Indicates the
position of all the ships as well which parts of space are empty.
- SIZE: A final attribute to indicate how large the galaxy is (10 for this
game).
- MAX_OPPONENTS: A final attribute that indicates the maximum number of
computer players in the game (3 for this assignment).
- You will also want to track which starship is controlled by the human
player (a single instance of class StarShip) and as the ones that are
controlled by the computer (a list of references to class
StarShip).
Behaviors:
- Move ships from one sector to another (subject to their maximum range
which is one sector in this version of the game but it may change in the
next assignment so keep this in mind as you design your program!)
- Displaying the galaxy.
- Checking the current state of the galaxy, determine if there are any
actions that the program has to do or what actions are available to the
players at that point in time. You will likely have to implement many
methods to handle all these cases e.g., You will need a method to check if
the player is able attack any other ships this turn - you can do this by
checking if any of the adjacent squares contain a computer-controlled ship.
- StarShip: For this game there will be four instances of this
class: 3 that are controlled by the computer and 1 that is controlled by the
human player.
Attributes:
- appearance: a character is used to store the appearance of the ship: a
'H' for the human-player's ship and a 'C' for the computer's ships.
- hullValue: an integer that stores the current hull integrity (number of
points) with a starting value of 400 each time that the game runs.
- shieldValue: an integer that stores the current shield strength (number
of points). The starting value = (starting hull value) * 10 Note: the
ratio of the shield points to the hull value may change in the next version
of the game.
- current coordinates: the row/column coordinates of the ship in the
galaxy.
- maxRange: an integer value that indicates the maximum number of sectors
that a ship may move each turn. For this version of the game it is one but
again this may change in the next version.
Behaviors:
- Determining the damage inflicted from the phasers when attacking another
ship. (Remember it must a randomly derived integer value in the range of 80
- 800 inclusive of both end points).
- The ability to absorb the damage inflicted by other starships. When one
ship is attacked by another ship, the second ship randomly generates a
damage value for the phasers. The first ship must take this value and
determine how much the shields and hull values get reduced. (Read the last
section of this assignment description on features that may added on for
Assignment 5 - otherwise you may have to totally rewrite this method when
you get to the next assignment!)
- Determine the current state of the ship e.g., Is it true or false that
the shields are down? Is the ship destroyed?
- Display the current state (shield strength and hull value) of the human
player's ship after the attack phase (see Figure 7).
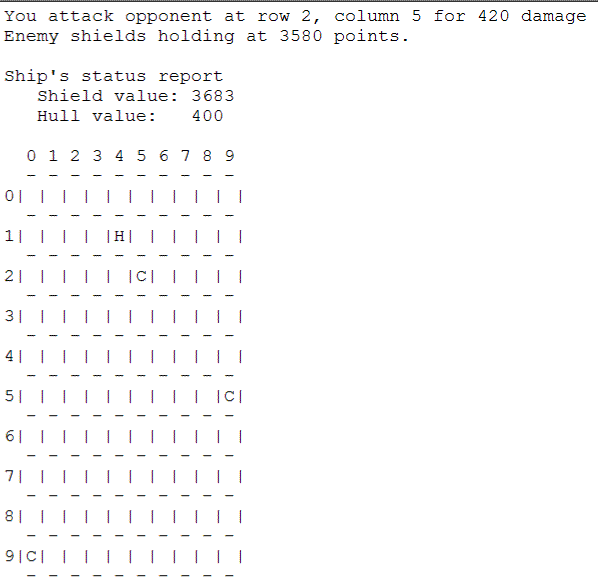 |
Figure 7: Status
report of the player's ship after the attack phase is done. |
- CommandProcessor: This class is responsible for getting input
from the user and for displaying the menus of options that are available at
a particular point in the game. Methods of this class take different
actions based on the user's input by invoking the methods of the other
classes.
Attributes:
- An instance of class Galaxy.
- The current menu option selected by the user.
Behaviors:
- Display the movement menu during the movement phase (see Figure 8).
The location of the numeric keys on the computer keypad determine the
direction of movement e.g., '7' performs an upward and leftwards movement.
Entering a value of zero will invoke a hidden cheat menu (see Figure 9) that
provides two options: (1) The ability to toggle cheat mode on/off. When
activated cheat mode will make the player's ship invulnerable to attack. (2)
The ability to toggle debug mode on/off. When the program is running in
debug mode debugging messages will appear onscreen that show the current
state of the program e.g., method names as they are being called, the
contents of different variables at different points in the program etc. The
exact content of the debugging messages are left up to your discretion but
you should use them to help you test and debug your program. See Figure 10
for an example of the debugging messages. The program should indicate each
turn when it is in cheat or debug mode.
- Display the attack menu during the attack phase (Figure 11). The
selection of the direction of attack is performed in a fashion that's
similar to selecting the direction of attack. Also the player should be able
to either quit the game or invoke the cheat menu from the attack menu.
- Note: With both menus if the user enters a negative number then the
program will immediately end and display some sort of parting end game
message (Figure 12). The pass option means that the player doesn't want to
do anything during that turn (the ship doesn't move during the move phase or
the person's ship doesn't attack during the attack phase - although any
adjacent computer-controlled opponents will still attack the player's
ship). Also note that as the program ends, a check must be performed to
determine if the player won or lost the game. If so then the end message
must indicate that either the person won or lost the game.
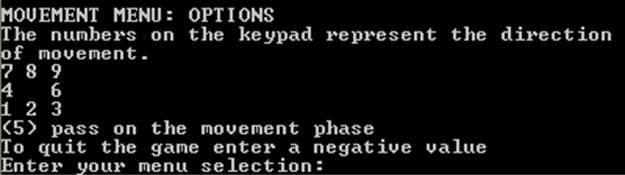 |
Figure 8: Movement
menu |
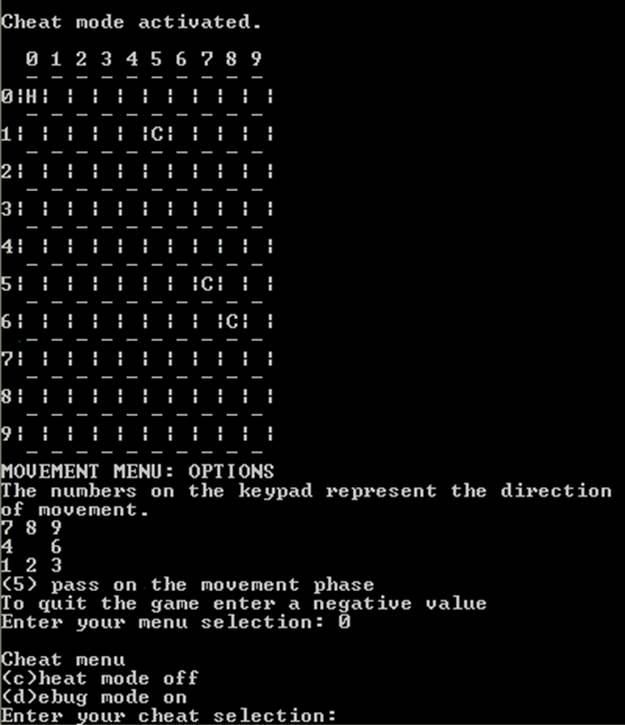 |
Figure 9: Cheat menu |
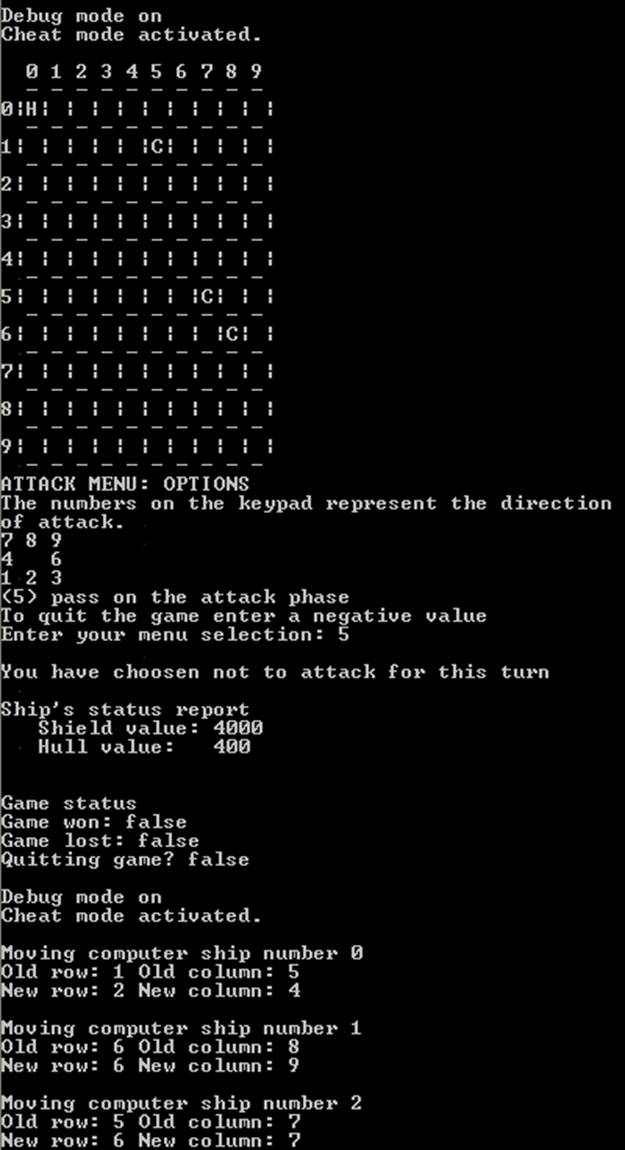 |
Figure 10: Example debugging
messages |
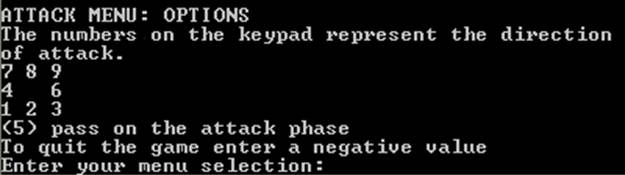 |
Figure 11: Attack
menu |
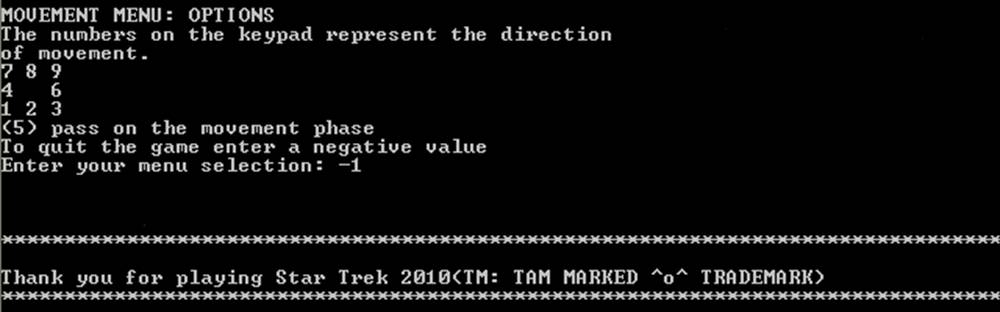 |
Figure 12: The end
game message if the player quits the program at any time. |
In addition your program must include my GameStatus class (or you write an
equivalent class yourself).
class GameStatus
{
/* Required attributes */
public static boolean debugModeOn;
public static boolean cheatModeOn;
/* You may also want to add two optional additional static
attributes */
public static boolean gameWon;
public static boolean gameLost;
}
Required attributes.
- debugModeOn: Indicates whether debugging statements show up as the
program is run. The exact content of the debugging messages is up to you
(see Figure 11 for some example output). Generally the messages that are
displayed onscreen are used to help the programmer determine where bugs are
and how to fix them e.g., when moving a starship your debugging messages may
display the current row/column coordinates as well as the coordinates of
where the ship is supposed to go. If this attribute is set to true then the
debugging messages appear. If the attribute is set to false then no
debugging message appear.
- cheatModeOn: Cheat mode will make the player controlled starship
invulnerable. It cannot be damaged by the enemies' phasers. Setting this
attribute to true turns on cheat mode while setting it to false turns off
cheat mode.
Optional attributes.
- gameWon: This attribute is set to true when the win game condition (see
above) is reached.
- gameLost: This attribute is set to true when the game is lost (see
above).
- (It is possible for the player to quit the game without either the game
win or game lost condition being met).
Of course if one these optional attributes
is true then the other cannot be true. They can be used in different parts of
your program to determine if it is time to end the game.
Submitting your work:
- Assignments (source code/'dot-java' files) must be electronically submitted
according to [the assignment submission requirements].
- As a reminder, you are not allowed to work in groups for this class.
Copying the work of another student will be
regarded as academic misconduct (cheating). For additional details about what
is and is not okay for this class please refer to the following [link].
- Before you submit your assignment to help make sure that you haven't
missed anything here is a [checklist] of items to be used in marking.
External libraries that can be used
- Libraries that allow for text-based (console) input and output.
- Libraries that will randomly generate numbers (e.g., class Random or class
Math).
Resources for further background information
It's not mandatory that you have to study the
contents of these websites in order to complete the assignment but you might
find the background information interesting (especially the long and detailed
explanation of how phaser technology is supposed to work).
-
|
-
To find out when your favorite episode
will air on local TV!: www.spacecast.com
|
-
|
-
A few sites in case you are really bored
and have nothing to do.
|
|
If you have no prior knowledge of the
Star Trek universe and want a little background information.
|
|
|
|
How do phasers work (long and short
explanations).
|
|
|
|
A not so academic comparison of
'Trekker' vs. 'Trekkie'. |
|
http://www.fortunecity.com/roswell/seance/134/lists/list15.html |
|
|
Future add-on that may apply for Assignment 5 (if the next assignment
extends the current one):
These are some things to keep in mind as you design this program because if
you make your program too specific for this assignment without considering
possible future extensions then you may have to rewrite major sections of your
Assignment 4 code.
- Travel is possible at faster than warp speed (thus extending their
movement range each turn).
- Cloaking devices have been invented (the invisibility that provides a
'first strike' capability).
- Opponents can adapt their shields to your weapon attacks (so your phasers
do less and less damage to them over time).
- Weapons have been developed that can penetrate shields (and directly
damage a ship's hull).
- Ships equipped with regenerative shielding (each turn the shields will
increase in points).
1 Note: The use of the Star Trek ©
trademark was for educational purposes only and not meant as a copy write
challenge.