CPSC 217: Pre-Mini-Assignment 1
Due at 4 PM. For assignment due dates see the
main schedule on the course webpage.
New Concepts to be applied for the assignment
- Learning how to create a complete
Python program from the ground up using: variables, getting input, displaying output, formatting output using escape codes &
format specifiers, using common operators. These things were covered in the
"introduction to programming lectures" and the respective
tutorials so you need to go through this material before starting the
assignment.
- If you want credit for your work: Do not use any
pre-created functions/methods unless you are given explicit permission to do
so. [Generic list of
allowable library functions]
Synopsis:
Write a python program that will perform calculations
similar to how your term grade with be determined for this class. To reduce the
need for implementing similar features over and over your assignment won't
include as many grading components. Although it's not identical to what's
used this term but similar enough to give you a good idea how your term grade
point will be calculated as well as allow you to apply many basic programming
tools.
- The program starts by prompting for the
grade point for each of these components (assignments and
examinations) one-at-a-time (refer to
Figure 1).
- Error checking that inputs are within range is not needed in this program because you
haven't been taught 'branching' yet. (This means that you may assume
that the user enters a value with the correct range)
- Error prevention: However it would be
a good idea to specify in the prompts to the user what is the valid
range of values (0 - 4.0 for assignments, 0 - 4.3 for the examinations).
- Also your program doesn't have to check for invalid types of
information being entered (e.g. the user enters a string instead of
numbers for a grade point) because you won't have learned "exception
handling" until much later in the term.
- Implementing this form of error handling won't be a violation of
using pre-created code because the exception handling model in python
does not employ pre-created functions.
- Next the program calculates and displays output: the weighted grade
points for each the assignments, examinations along with the original
grades and the term grade point (refer to Figure
2).
- Calculating a weighted grade: The general formula
for calculating a weighted term grade was covered in the first set of
lecture notes dealing with administrative issues such as grading but the
basics are simple. The weighted grade for a component (whether that grade is
a GPA, percentage or raw score)
= (grade for the component) * (weight of that
component). This product is calculated for each
component and the weighted component grades are summed to yield a term
grade.
- Grade point example:. You score 3.7 on a grading
component worth 70% and 4.1 on the other grading component worth 30%, the
term GPA = (3.7* 0.7)+(4.1*0.3) = 2.59 + 1.23 =
3.82 (should be a letter grade of at least an
A-).
- Percent score example (percentages aren't used in
the assignment nor in the calculation of your actual term grade but it's
included to help you understand how to calculate a weighted term grade): You
score 80% on a grading component worth 70% and 100% on the other grading
component worth 30%, the term percent = (80*
0.7)+(100*0.3) = 56 + 30 = 86% (to determine
your letter grade you have to look at instructor's specific cut off scale
which is one reason why this instructor uses grade points rather than
percentages - it's not self evident what the term letter will be if
percentages are used - contrary to what some students may believe this
university does not impose a required set of cut offs for percentage to
letter grade mapping).
- Components of the term grade for the program you
are to write for this assignment:
Component |
Proportion of term
grade point (weight) |
A1 |
15% |
A2 |
15 |
A3 |
20 |
Midterm exam |
20 |
Final exam |
30 |
Total |
100% |
- Normally you should test your program by hand tracing different inputs
and comparing the results with the output of your program. Since that might
be time consuming for this assignment you can check your results by entering
sample results with the grade calculator spreadsheet that I've customized
for this assignment: [Pre-mini-assignment1
grade calculator]
- Formatting your output
- Each grade point will be display with one place of precision
(specified by the correct use of the format specifier, although there
are other ways to do this in python this approach is standard with many
languages so you need to do it this way in order to get credit),
precision is the number of digits right of the decimal point. (If you still don't
know what this means here's
[an article] explaining the term).
- Tabs (each one must specified with the appropriate escape code) will separate
each piece of information (label or individual grade).
Figure3 includes an annotated version of the output
of my solution specifying the location of the escape codes.
- <Assignments:> <tab> <GPA for A1> <tab> <GPA
for A2> <tab> <GPA for A3>
- <Examinations:> <tab>
<GPA for the midterm> <tab> <GPA for the final
exam>
- <Weighted assignments/exams> <tab>
<Weighted assignment GPA> <tab> <Weighted exam GPA>
- <Term GPA:> <tab> <Actual
GPA for the term>
- The angled brackets do not actually appear in the
output, they are only used to separate different pieces of information. The
spaces between the fields shown above are only included for readability and do
not appear in the final output (e.g. there is only a tab between A1 & A2 not a
space and a tab).
- The column for the labels ("Full assignments",
"Examinations" etc. must be right aligned using a correct
format specifier (field width) so that the colons line up
on top of each other). Alternative approaches (e.g. typing a bunch of spaces
between the quotes in the print
function) will be awarded no credit. See the lecture material
covering format specifiers on how to align a column of output
("Introduction to programming Part 2").
Figure 4 includes an annotated
output of the my sample solution specifying where the format specifiers are
used to align the output.
Explicit reminder to be awarded credit for the formatting output:
- Format specifiers must be used when a column width or column alignment is specified
(e.g. right or left alignment).
- Also format specifiers must be used to specify
the number of places of precision for floating point output (can have a
fractional or value .
- Other formatting effects (e.g. tabs, newlines) must be implemented using escape codes (e.g. add tabs between columns).
Figures showing the program getting input from the
user and displaying program output
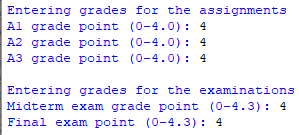
Figure 1: Getting input
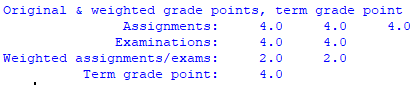
Figure 2: Output of the original grades, the
weighted grades and the term grade point.
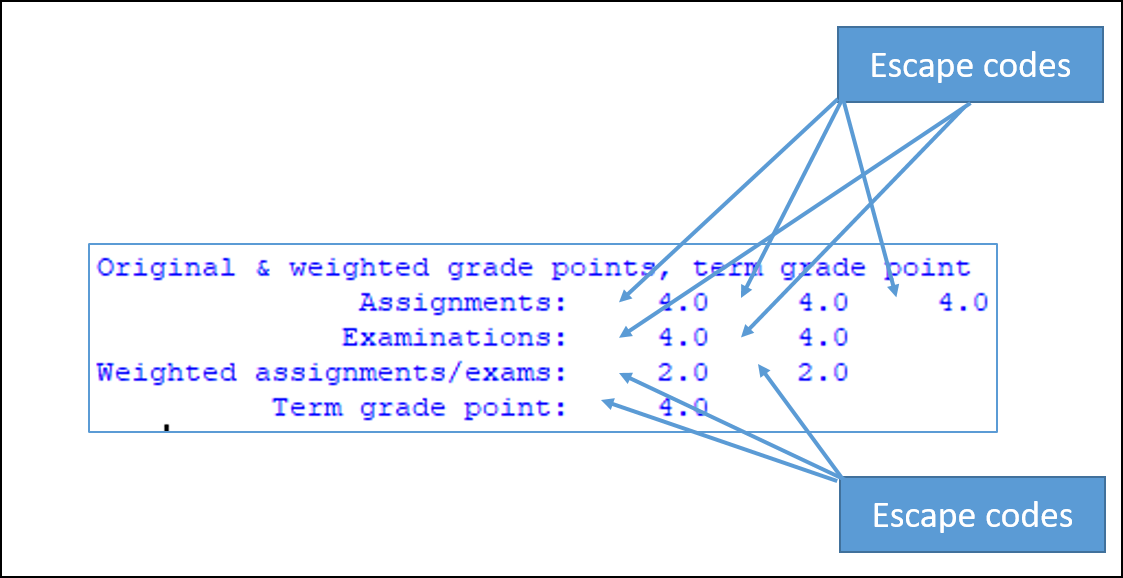
Figure 3: Output of a sample solution
annotated with the location of where escape codes format the output.
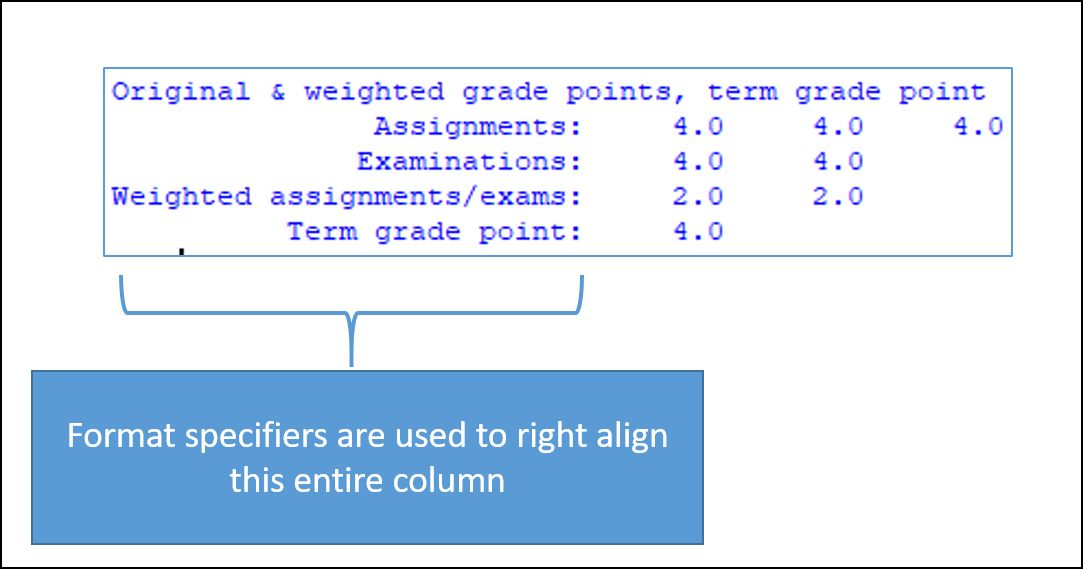
Figure 4: Format specifiers
used to align a column
How to do determine 'how you did' on
an assignment?
Depending upon the number of assignments the grading for previous assignments
may not be released before the next one comes due. Although this can be
problematic in assignments where marking is not specified in detail and marking
is fairly subjective this should not be a problem this term. You will not only
be given a breakdown in how grades will be allocated by program feature in the
assignment description (mini-assignments) or a grading Excel sheet will be
provided. Also, when writing computer programs for this class you should, if you
approach things correctly, know as you submit your work roughly what your
assignment grade will be:
Program functionality (implementing working program features)
- Test your program: Because the
assignment description (along with required features) is posted ahead of
time if you test your program thoroughly before submitting the final
version then you should get a pretty clear idea of "how you will do".
Normally you should "hand
trace" your program and compare the results of running your program. The
calculations are a bit long in this case so for this assignment You can use
the [grade calculator spreadsheet]
that was tailored for this assignment to compare results.
Style and documentation (non-functional assignment requirements)
- To keep the marker's workload reasonable and to reduce marking time
unless otherwise specified in the description these things will not
factor into the grading of mini-assignments.
Although it won't affect your grade for mini-assignments you should still
practice applying good style in your solution as well as writing
documentation. It will keep your skills for the full assignments (when you will
be graded on these things) and get you used to having good habits.
- Naming conventions: You should employ good naming conventions for
identifiers (variables and constants) as covered in the "Introduction to
programming" section of the course.
- Defining and using named constants. Although this
assignment does not specifically require the use of named constants later
assignments will do so and it would be good practice to start employing this
practice now.
- Python documentation: This should be specified
in the header of the program (very top of your program in the form of Python documentation).
The basics of documentation were covered in the "Intro to programming"
section of the course. Later sections may include additional details.
-
Identifying information:
All assignments should include contact information (full name, student ID
number and tutorial section [Here's
a
Link if you don't know how to find this information]) at the very top of your program.
- Program version.
(version date or version number).
- Under the version you
should specify which assignment features were implemented in that version.
An example covering the use of program versioning and documentation was
covered in the "Introduction to programming" lectures.
-
Any program limitations or weaknesses.
- [As mentioned] your prompts to the user to enter the
required information must be clear to the user as to what should and
should not be entered.
- In general: The program output and the program code itself
(python program) must be properly aligned and formatted including whitespace
as needed to group related parts and separate sections (which again was
covered in the "Intro to programming" lectures of the course.
Additional requirements in the formatting of output may be included in the "Functional
requirements" section of the assignment.
Optional python feature that you can use
The calculations for the weighted grade points for each
major course component can be long but python won't allow you to simply hit
enter and continue a single python instruction on the next line. (You must use the line
continuation character (back slash) properly). Here's a [simple
example] illustrating how it works.
-
What to submit (remote
learning version): If your submission won't run using Python 3.x on any
computer then it won't be awarded credit. It's up to you if you wish use
the graphical program builder other than IDLE (or another development
environment rather than a simple text editor) to write/run your programs but
if you do you submit your program in the form of text ".py"
file or files. Windows users: take care that
you don't accidentally submit a shortcut to a file instead of the actual
file. (Check the file name and compare the file size to your
original file. Simply downloading the shortcut file as a test won't work
because that shortcut will work on your computer but not on anyone else's
machine and this may mean your work won't be graded.)
-
Naming the file containing your program: You must save your program in a file called "grader.py".
Failing to use this exact name will affect your grade by -0.2 GPA.
-
Late assignments or
components of assignments/Assignment extensions: Normally due dates
are strict and extensions require documentation. Due to the likelihood of
widespread Covid related illnesses late penalties will not be applied this
semester. But if you do submit something after the due date/time then you
should email your marker (TA
in the tutorial that you are officially registered) so that person will
know to look for it in D2L. Also if are submitting too many graded components late however this
doesn't bode well for your performance in this course so you may be referred
to the associate dean of undergraduate students (faculty of science) and she
may contact you regarding your course work.
-
How you will be graded
for full assignments. The grading for most mini-assignments is
usually quite simple and the information about mark breakdown is often
included right in the assignment description. Because this is your first
grade component and it's a bit more detailed than most mini-assignments this
one
will include a separate [marking checklist]. Besides seeing your grade point
in D2L you can also see the detailed feedback that your TA will enter for
each student. You can access the grading sheet in D2L under Assessments->Dropbox and
then clicking on the appropriate assignment link. If you still cannot find
the grading sheet then here is a [help
link]
-
Questions or concerns about grades after they have been released:
Assignments will be marked by your tutorial instructor (the "Teaching
Assistant" or "TA") for your
tutorial section. When you have questions about marking this is the first
person that you should be directing your questions towards.
If you still have question after you have talked to your TA, then you can
of course talk to your course
(lecture) instructor but
please indicate in your email that you first contacted your TA before going
into your concerns.
Collaboration:
Assignments must reflect individual work;
group work is not allowed in this class nor can you copy the work of
others. Some "do nots" for your solution:
don't publically post
it, don't email it out, don't show it to other students. For more detailed information as to what constitutes academic
misconduct (i.e., cheating) for this course please read the following [link].
Method of submission:
You are to submit your assignment using D2L [help
link].
Make sure that you [check
the contents of your submitted files]
(e.g., is the file okay or was it corrupted, is it the correct version
etc.). It's your responsibility to do this! (Make sure that you submit your
assignment with enough time before it comes due for you to do a check). If
don't check and there were problems with the submission then you should not
expect that you can "learn your lesson" and simply resubmit.
-
Do not use compression utilities (such as zip) or archiving utilities
(such as tar) otherwise your submission may not be marked. The space savings
in D2L is not worth the extra time required by the marker to process each
submission.
-
How often can you submit: Multiple
submissions are allowed for this assignment:However
only the latest file submitted is the one that will be marked, everything
else will be ignored (because it is not fair to your marker to sort through
multiple versions of your files). For that reason you should always strive
to submit your work on time (so the marker doesn't have a newer version to
check after the last version was already graded - beyond that it's not a
good idea to fall behind in this class).
- What to submit:
Python programs only (file name ends in
.py)
Any other type of file will be rejected by D2L.
(Waived for
fall 2022),
Late submissions
for full assignments when there is no extension granted:
Again let
(TA
in the tutorial that you are officially registered) know if you do
submit late.
Make sure you give yourself enough time to complete the submission process so
you don't get cut off by D2L's deadline (or your submission will be
automatically flagged as
late by D2L and it will be graded appropriately)..
Submission received: |
On time |
Hours late : >0 and <=24 |
Hours
late: >24
and <=48 |
Penalty: |
None |
-1
GPA |
-No credit
(not accepted)
|
Unless otherwise told you are to write the code yourself and not use any
pre-created functions (or methods). For most assignments the usual
acceptable functions include:
print(),
input()
and the 'conversion' functions such as
int(),
float(),
str(). Look at the particular assignment description for a list of other
functions/methods that you are allowed to use and still get credit in an assignment
submission. If it's not listed then you should assume that you won't be able
use the function and still be awarded credit.