CPSC 217: Assignment 1
Due at 4 PM. For assignment due dates see the
main schedule on the course webpage.
Assignment difficulty
Students may find assignments more challenging than
they first thought. It's best to start work as early as possible. Tips in the
very first lecture were
provided but here's two reminders: 1) work through the lecture and tutorial
material before looking in detail at the assignments 2) start work as soon as
possible. If you find you cannot complete an assignment before the due date then
you will not be granted an extension.
Note: it is not sufficient to just implement a working program
and expect full credit. This is so you implement your solution in the correct
way using good design principles and you apply the necessary concepts. Even
if your program is fully working and the program is not designed or implemented
as specified in the assignment description (e.g. poor variable names used, named
constants not used when they should have employed etc.) then you will not be
awarded full credit.
For some assignments your grade may be greatly reduced. For example, in all the
later assignments you need to employ functional decomposition and do
so in a the proper specified manner. If you do not do this then your maximum
assignment grade point could be as low as 1.0 GPA even with a fully working
program. Although the earlier assignments will not have marking requirements
which are quite as strict
take
care to read the specific details provided in each assignment which is provided
under the "Marking and grading" section
of each 'full' assignment. The marking of the mini-assignments will focus on
program functionality.
New Concepts to be applied for the assignment
- Learning how to create a complete
Python program from the ground up using: variables, documentation,
getting input, displaying output, formatting output using escape codes &
format specifiers, using common operators. These things were covered in the
"introduction to programming lectures" and the respective
tutorials.
- If you want credit for your work: Do not use any
pre-created functions/methods unless you are given explicit permission to do
so. [Generic list of allowable
pre-created code in the form of python libraries]
Creating your first Python program
Write a python program that will perform the actual calculations used to
calculate your term grade. It will start by prompting for the
grade point (0 - 4.3)1 for each of these components (assignments and
mini-assignments) one-at-a-time.
1 During a particular semester only some
assignments will allow for a bonus (grade point greater than 4.0) but you don't
have to account for these issues in your prompts to the user.
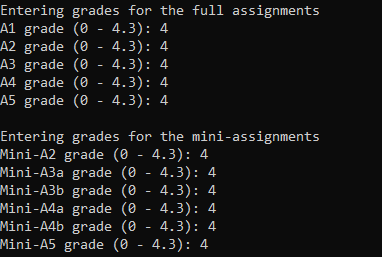
Figure 1: Getting input
Your program does not have to error check the user
actually entered a value within this range because you will not yet have yet
learned how to do this yet. Just assume that the user enters a value within the
correct range. (Note Also your program doesn't have to check for invalid types of
information being entered (e.g. the user enters a string instead of numbers for
a grade point). You should however document these problems as current program
limitations (see the "non
functional requirements" section).
After the grades for all the components have been
entered the program will calculate
and display
the weighted grade points for each of the full assignments, mini-assignments and
the term grade point. It will display the original grades entered by the user
along with the weighted grades and the term grade.

Figure 2: Output of the original grades, the
weighted grades and the term grade point.
Fractional values should be displayed with one place of precision,
precision is the number of digits after the decimal point. (If you still don't
know what this means here's
an article explaining these terms). The precision must be specified with a
correct format specifier.
Here's the format of the output (original grades,
weighted grade points and the term grade point):
- <Full assignments:> <tab> <GPA for A1> <tab> <GPA
for A2> <tab> <GPA for A3> <tab> <GPA for A4>
<tab> <GPA for A5>
- <Mini assignments:> <tab> <GPA for mini-A2> <tab> <GPA for mini-A3a> <tab> <GPA for
mini-A3b> <tab> <GPA for mini-A4a>
<tab> <GPA for mini-A4b> <tab> <GPA for mini-A5>
- <Weighted full assignments> <tab> <Weighted
GPA for all the full assignments>
- <Weighted mini-assignments> <tab> <Weighted
GPA for all the mini assignments>
- <Term GPA:> <tab> <Term GPA>
The angled brackets do not actually appear in the
output, they are only used to separate different pieces of information. The
spaces between the fields shown above are only included for readability and do
not appear in the final output (e.g. there is only a tab between A1 & A2 not a
space and a tab.
Here's how you are to produce the specified format:
- The column for the labels ("Full assignments",
"Mini assignments" etc. must be right aligned using a correct
format specifier (field width) so that the colons line up
on top of each other). Alternative approaches (e.g. typing a bunch of spaces
in the print
function) will be awarded no credit.
- As stated, only one place of precision will be
used for each grade using the correct format specifier.
Explicit reminder to get credit for formatting output:
- Format specifiers must be used when a column width or column alignment is specified
(e.g. right or left alignment).
- Also format specifiers must be used to specify
the number of places of precision.
- Other formatting effects (e.g. tabs, newlines)
must be implemented using escape codes (e.g. add tabs between columns).
Testing your program:
Normally you should "hand
trace" your program and compare the results of running your program. You can use
the [grade calculator spreadsheet] for
this assignment to compare results.
- Naming conventions: You should employ good naming conventions for
identifiers (variables and constants) as covered in the "Intro to
programming" section of the course.
- Python documentation: contact information (your name,
student identification number and tutorial number). This should be specified
in the header of the program (very top of your program in the form of Python documentation).
-
Identifying information:
All assignments should include contact information (full name, student ID
number and tutorial section) at the very top of your program.
- Program version.
- Under the version you
should specify which assignment features were implemented in that version.
-
Any program limitations or weaknesses.
- If you don't know how
to document a program then refer to the "Intro
to programming" section of the course.
- Your prompts to the user to enter the
required information should be clear to the user as to what should and
should not be entered.
- The program output and the program code itself
(python program) must be properly aligned and formatted including whitespace
as needed to group related parts and separate sections (which again was
covered in the "Intro to programming" lectures of the course.
Having at least one violation in one of the above
categories will result in -0.1 penalty to marking. Multiple violations in one
category still results in a single penalty (e.g. 3 bad variable names will
still result in a -0.1 penalty). However violations between categories
will result in cumulative penalties (e.g. a program that includes poor variable
names and no documentation of features and no documentation of weaknesses will receive a -0.3 penalty).
-
What to submit:
In a typical semester submitted programs must run on the computer science network running Python 3.x. If you
write you code in the lab and work remotely using a remote login program
such as Putty or SSH then you should be okay (assuming you don't login to a
non-Linux computer). If you choose to install Python on your own computer
then it is your responsibility to ensure that your program will run properly
here. Remote learning version: If it won't run using Python 3.x on any
computer then it
won't be awarded credit. It's up to you if you wish use the graphical
program builder IDLE (or another development environment rather than a
simple text editor) to write/run your programs but if you do you submit your program in the form of
text ".py" file or files.
-
Late assignments or components of
assignments: will not be accepted for marking without approval for an
extension beforehand. Alternate submission mechanisms (non exhaustive list
of examples: email, uploads to cloud-based systems such as Google drive,
time-stamps, TA memories) cannot be used as alternatives if you have
forgotten to submit work or otherwise have not properly submitted into D2L.
Only files submitted into D2L by the due date is what will be
marked, everything else will be awarded no credit. The final cut off
date after which full assignments will not be accepted is after the
maximum progressive
penalty (listed below) can be applied. The final cut off date for
mini-assignments is the due date because no late submissions are allowed for
these smaller programs.
-
Extensions
may be granted for reasonable cases by the course instructor with the
receipt of the appropriate documentation (e.g., a sworn declaration with a
commissioner of oaths). Typical
examples of reasonable cases for an extension include: illness or a death in
the family. Example cases where extensions will not be granted include situations
that are typical of student life: having multiple due dates, work
commitments etc. Tutorial instructors (TAs) will not be able to provide
extension on their own and must receive permission from the course
instructor first.
If you request an extension from me let me know the name of your tutorial
instructor and the tutorial number because the markers won't accept
late submissions without directly getting an email from me.
-
How you will be graded for full assignments. As mentioned, as well as being marked
on whether "your program works" you will also be marked on non-functional
requirements such as style and documentation. Consequently this assignment
will include a separate [marking checklist].
Besides seeing your grade point in D2L you can also see the detailed
feedback that your TA will enter for each student. You can access the
grading sheet in D2L under Assessments->Dropbox and then clicking on the
appropriate assignment link. If you still cannot find the grading sheet then
here is a [help link]
-
Questions about grading afterward:
Assignments will be marked by your tutorial instructor (the "Teaching
Assistant" or "TA") for your
tutorial section. When you have questions about marking this is the first
person that you should be directing your questions towards. If you still
have question after you have talked to your TA, then you can talk to your
course (lecture) instructor but please indicate in your email that you first
contacted your TA.
Method of submission:
You are to submit your assignment using D2L [help
link].
Make sure that you [check
the contents of your submitted files]
(e.g., is the file okay or was it corrupted, is it the correct version
etc.). It's your responsibility to do this! (Make sure that you submit your
assignment with enough time before it comes due for you to do a check). If
don't check and there were problems with the submission then you should not
expect that you can "learn your lesson" and simply resubmit.
-
Multiple submissions are allowed for this assignment (all versions are kept
in D2L): You can (and really should) submit work as many times as you wish
before the due date.
However only the latest version of all the files is what will be marked,
everything else will be ignored (because it is not fair to your marker to
sort through multiple versions of your files).
-
Do
not use compression utilities (such as zip) or archiving utilities (such as
tar) otherwise your submission may not be marked. The space savings in D2L
is not worth the extra time required by the marker to process each
submission.
Late submissions
for full assignments when there is no extension granted:
Make sure you give yourself enough time to complete the submission process so
you don't get cut off by D2L's deadline (or your submission will be
automatically flagged as
late by D2L and it will be graded appropriately)..
Submission received: |
On time |
Hours late : >0 and <=24 |
Hours
late: >24
and <=48 |
Hours
late: >48
and <=72 |
Hours
late: >72
and <=96 |
Hours
late: >96 |
Penalty: |
None |
-1
GPA |
-2
GPA |
-3
GPA |
-4
GPA |
No credit
(not accepted) |
Collaboration:
Assignments must reflect individual work;
group work is not allowed in this class nor can you copy the work of
others. Some "do nots" for your solution:
don't publically post
it, don't email it out, don't show it to other students. For more detailed information as to what constitutes academic
misconduct (i.e., cheating) for this course please read the following [link].
Unless otherwise told you are to write the code yourself and not use any
pre-created functions (or methods). For most assignments the usual
acceptable functions include:
print(),
input()
and the 'conversion' functions such as
int(),
float(),
str(). Look at the particular assignment description for a list of other
functions/methods that you are allowed to use and still get credit in an assignment
submission. If it's not listed then you should assume that you won't be able
use the function and still be awarded credit.