CPSC 231: Mini Assignment 4B
Due
Wednesday Nov 23
at 4 PM
New Concepts to be applied for the assignment
- Learning how to get a program to react to GUI events (button press)
- Drawing simple graphics and configuring graphical properties during
program run-time
- Both parts must be implemented using the TkInter library module
of Python
Part I: Creating a simple graphical user interface
Modify the existing code in the program 'button_events.py'
program to that a text message appears onscreen when the user presses
the button. (You can use the print() function to
display the message).
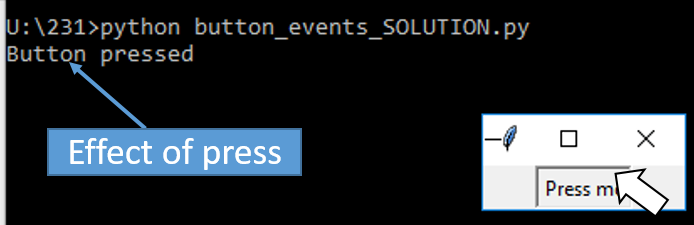
Part II: Drawing simple graphics
Modify the starting code in the program 'graphics.py'
program. In function drawShape(),
the program draws a rectangle bounded on the top LHS point (100,200) and the
bottom RHS point(300,300) coloured yellow. Use the information from the
getBounds() function to get the coordinates for the
top LHS point as well as the size of the rectangle. The coordinates of the
bottom RHS point will be equal to the (x,y) coordinates of the top LHS point
plus the size. (Your solution doesn't have to error check the bounds make sense
although you can do so for extra practice). Example input: Top LHS
Point(100,200) size = 200 yields bottom RHS point (100+200,200+200) or
(300,400). Your modifications will allow the position and bounds of the
rectangle to be determined at run time. Also modify the program so the fill
color won't be fixed but instead be based on the return value of the
getFillColor() function. (Again your program
doesn't have to check that the color entered is one of the allowable fill colors
although you can do it for extra practice):
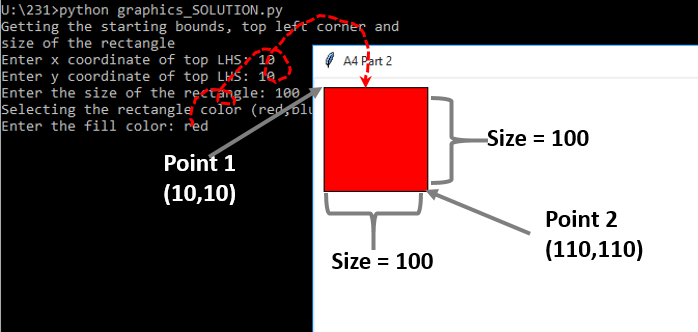
[TA
Marking spreadsheet]
Points to keep in mind:
- Due time: All assignments are due at 4 PM on the
due dates listed on the course web page. Late assignments or components
of assignments will not be accepted for marking without approval for an
extension beforehand. What you have submitted in D2L as of the due date is
what will be marked.
- Extensions may be granted for reasonable cases by the course
instructor with the receipt of the appropriate documentation (e.g., a
doctor's note). Typical examples of reasonable cases for an extension
include: illness or a death in the family. Cases where extensions will not
be granted include situations that are typical of student life: having
multiple due dates, work commitments etc. Tutorial instructors (TA's) will
not be able to provide extension on their own and must receive permission
from the course instructor first. (Note: Forgetting to hand your assignment
or a component of your assignment in does not constitute a sufficient reason
for handing your assignment late).
- Method of submission: You are to submit your assignment using D2L
[help
link]. Make sure that you [check
the contents of your submitted files] (e.g., is the file okay or was it
corrupted, is it the correct version etc.). It's your responsibility to do
this! (Make sure that your submit your assignment with enough time before it
comes due for you to do a check).
- Identifying information: All assignments should include contact
information (full name and student ID number) at the very top of your
program in the class where the 'main()'
function/method resides.
- Collaboration: Assignments must reflect individual work,
group work is not allowed in this class nor can you copy the work of
others. For more detailed information as to what constitutes academic
misconduct (i.e., cheating) for this course please read the following [link].
- Execution: programs must run on the computer science network
running Python 3.x. If you write you code in the lab and work remotely using
a remote login program such as Putty or SSH. If you choose to install Python
on your own computer then it is your responsibility to ensure that your
program will run properly here. It's not recommended that you use an IDE for
writing your programs but if you use one then make sure that you submit your
program in the form of text ".py" file or files
- Use of pre-created Python libraries: unless otherwise told you
are to write the code yourself and not use any pre-created functions from
the Python libraries. For this assignment the usual acceptable functions include:
print(), input(),
sys.stdout.write(), str(),
float(), int() as
well as the capabilities of the TkInter library module and if you wish
assert().
D2L configuration:
- You can and should submit many times before the due date. D2L will
simply overwrite previous submissions with newer ones.
- Needed for this assignment if you wish to submit separate programs
for both parts: Only one file can be submitted per assignment so you
should combine multiple files into one file using a program such as zip.
Marking:
- Assignments will be marked by your tutorial instructor (the "Teaching
Assistant" or "TA"). When you have questions about marking this is the first
person that you should be directing your questions towards. If you still
have question after you have talked to your TA then you can talk to your
course (lecture) instructor.
Starting code:
The starting code can be found in UNIX under:
/home/231/assignments/mini_assignment4b/code
You need to use this starting code in your own solution, just make the
necessary modifications in order to fulfill the above requirements.