Due
Wed April 13
at 4 PM
A4: JavaScript Web Page Programming
As usual all students begin with a grade point of zero on this assignment.
Implementing the features described below will earn you grade points equal to
the value specified in the brackets.
Part I: Webpage with html tags
Sample themes for the page include a personal page, a page your favorite organization or
group (sports team, charity, hobby etc.) Generally you are pretty free in your
choice of website theme. However it should be something that is appropriate for
a university assignment submission. (You should be able to apply a common-sense
filter as to what is and isn't allowed but if you are unsure then feel free to
ask!) To make sure that you comply with copyright laws the multi-media content
that you imbed in your document should either be images and videos that you have
made yourself (e.g., a picture that you took) or else material where the
license agreement allows for public use by others. When in doubt it's safer to
simply 'link' to an external website
rather than directly post the material yourself on your university page. If you don't know the
difference between imbedding an image or video vs. external links then apply the
following criteria. If the image or video resides in the 'public_html'
folder (or a sub-folder) then you have to worry about copyright (because you are
the one providing the content). If the image or video resides on another
computer web server (e.g., www.youtube.com)
then whoever posted the content must worry about copyright.
The university provides some information about the current state of copyright
issues for academic use: [Information
link here]
The structure for an example webpage is shown in Figure 1.

Figure 1: The
text is separated into a title, sections and section headings
- Title and headings (0.1 GPA):
The page should include a clear and obvious title (use font effects and/or a 'heading'
tag to make it stand out strongly from the rest of the page). See top of
Figure 1.
- Sections (0.2 GPA):
The main body of the website should consist of at least two sections (0.1
GPA) each of which should have a section heading which stands out from the
text (0.1 GPA) (see the 'Programming MS-Word' and 'Features' headings in Figure 1).
- Text formatting via html tags (0.1 GPA):
Use at least 3 of the following tags: bold, italics, strike-through,
underline at least once (for each tag) in the page.
A list (0.1 GPA):
either 'ordered' (numeric) or 'unordered' using a "list tag" must also be
included as part of the page.
Break content into separate lines (0.1
GPA): The 'break' tag should
be used as appropriate to separate lines.
Embedded content (0.2
GPA): include at least one
image or video that is locally hosted on the university web server. A better designed
(higher grade) web page will organize all
the content into it's own separate folder that is contained within your 'public_html'
folder.
External link or links (0.1
GPA): Use an 'href' to link to
an external website or websites.
Contact information (0.1
GPA): Use a 'mailto' tag to
allow visitors to send you an email. The destination address should be one
of your own emails (don't spam someone else).
Your webpage can be viewed online
(0.1 GPA):
Include the web address of your University of Calgary hosted webpage with
the rest of your D2L submission in a Word document. In order to get credits for this
feature it must be a real web address (i.e. begin with
https://webdisk.ucalgary.ca/)
Part II: Webpage augmented with JavaScript. In addition to getting marks for
the working JavaScript functionality (described below) this part of the submission
will be marked according to program-writing style and documentation requirements
that you have been taught in lecture.
Figure 2:
Graphical controls created using html tags
The website should allow visitors to enter
their contact information via text input fields: title, first & last name. There will
be two buttons: 'cancel' that clears all of the
input fields and 'confirm' that will take
information from these fields and send it via email (Figure 2 shows the
graphical controls with sample data).
Some webpage content is needed for this part of the assignment:
- Creating the 5 graphical controls (0.1 GPA):
The 5 controls shown in Figure 2 include two 'buttons'
and three 'text' controls. The controls are
created using html tags
- Label the controls (0.1 GPA): Type
the text into the webpage make sure that the 3 text controls are clearly and
presentably labeled 'Title',
'First Name', 'Last Name'.
In order to make the webpage interactive
JavaScript will be used to augment the webpage.
- Cancel button (0.3 GPA): clears the
3 text input controls
- Confirm button (0.1 GPA):
run email client via 'mailto' (to earn the 0.1
GPA the email address doesn't have to come from the text controls it just
has to send to some email).
- Email subject (0.1 GPA): Email subject: Subject line is correctly set to
the assignment number and must be exactly as follows "Assignment
4"
- Email body, salutation (0.1 GPA): Prefaces
the start of the email with the salutation 'Dear'
- Email body, title (0.2 GPA): Immediately follows the previous field
(salutation) with
the 'title' text input field. Half credit if
not properly capitalized (it would be "Sushi-Master" in the example shown in
Figure 2.
- Email body, first name (0.1 GPA):
Immediately follows the previous field with the first name text field.
- Email body, last name (0.1 GPA): Immediately follows the previous field
with the last name text field.
- Email recipient address (0.1 GPA): Some predetermined fixed
email address that
you own (don't have your website send spam email to someone else!).
Pressing the 'confirm' button with the data
shown in Figure 2 will run the email client configured for the webpage visitor's
computer as shown in Figure 3.
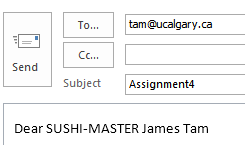
Figure 3:
Documentation requirements, Part II:
- Listing assignment features completed (0.4 GPA):
0.05 for each JavaScript feature correctly listed as completed or not completed
- Program versioning (0.1 GPA):
Evidence that some sort of versioning system was employed
Style requirements, Part II:
- Poor variable naming conventions (variable and function name):
-0.1 GPA penalty for any violations
- Each program level not indented by 4 spaces for each 'level':
-0.1 GPA penalty for any violations
- (Lack of necessary instructions may result in JavaScript functionality
marks getting missed in marking) as well as a style penalty
Page III: JavaScript implements a "times-table" multiplication program.
In addition to getting marks for
the working JavaScript functionality (described below) this part of the submission
will be marked according to program-writing style and documentation requirements
that you have been taught in lecture.
The JavaScript function automatically runs when your webpage is loaded into
the browser. Except for some information in the html part of the webpage to
automatically run the JavaScript function (when the content of the page is
loaded into the browser) everything for this part of the assignment should be
inside of a script tag. No other webpage content or html tags are needed. The program will calculate the multiplication times-tables for
any two products from 1 - 12 using a pair of nested loops. You will need to be
proficient writing programs employing branching (using ifs,
else if etc.) and not only basic loops but also
nested loops before starting this assignment. Also you must know the correct
terminology inside and out. If the assignment specifications don't appear to
make sense then it's probably because you don't properly understand a concept or
term.
Built in mathematical
functions of JavaScript cannot be used although of course you can and must use
mathematical operators such as assignment and multiplication. Each product will
be written to the webpage using the 'document.write()' method. To provide some
readability each product must be followed by a space. For example 1 x 6 yields:
1 2 3 4 5 6.
The products for each row should be a separate
line. Example: 2 x 3: (1x1, 1x2, 1x3, 2x1, 2x2, 2x3):
Columns ->
Rows 1 2 3
|||||||
vvvvvvv 2 4 6
- User input (0.05 GPA): using the
prompt() functions the program will get the two
numbers to be multiplied. The first specifies the number of rows in the
table, the second specifies the number of columns. In the last example above
(2 x 3) the table of multiplied values consist of 2 rows and 3 columns.
- Repeated prompts, rows (0.1 GPA):
Use a loop to error check the number of rows and repeatedly prompt until valid
value (1 - 12) is entered1.
- Repeated prompts, columns (0.1 GPA):
Use a loop to error check the number of columns and repeatedly prompt until valid
value (1 - 12) is entered1.
- Error message, rows (0.05 GPA):
Using a branch, displays an appropriate error message2 when the number of rows is
too low. The error check and error message must appear within the body of
the loop (second bullet above).
- Error message, rows (0.05 GPA):
Using a branch, displays an appropriate error message2 when the
number of rows is too high. The error check and error message must appear
within the body of the loop (second bullet above). The error message is different when the
value is too low vs. too high.
- Error message, columns (0.05 GPA):
Using a branch, displays an appropriate error message2 when the number of columns
is too low. The error check and error message must appear within the body of
the loop (third bullet above).
- Error message, columns (0.05 GPA):
Using a branch, displays an appropriate error message2 when the number of columns
is too high. The error check and error message must appear within the body
of the loop (third bullet above). The error message is different when the
value is too low vs. too high.
- Calculates the products, each row value x each column value (0.2 GPA):
Employs a nested loop to calculate the product of current row and column
values
- Displays results (0.2 GPA): Writes
the products to the webpage. It must appear in the fashion described (spaces
and newlines) otherwise at most only half marks are awarded for correct
results (your marker isn't required to decipher garbage output even if the
results are correctly generated).
1
As shown in Video 2, the repeated prompting for the rows is complete
before the program prompts for the number of columns. In other words the
value for the number of rows is valid before the program starts asking for
the number of columns. If the column value is outside the valid range the
program will repeatedly prompt for a value until a valid column value is entered.
Once a valid (row, column) pair has been entered the nested loops will
calculate the products and write each one to the document until all the
products have been calculated.
2 A good error message should not only tell
the user what went wrong but how to prevent the error from occurring again.
Documentation requirements, Part III:
- Listing assignment features completed (0.45 GPA):
0.05 for each feature correctly listed as completed or not completed
- Program versioning (0.1 GPA):
Evidence that some sort of versioning system was employed
Style requirements, Part III:
- Poor variable naming conventions (variable and function name):
-0.1 GPA penalty for any violations
- Each program level not indented by 4 spaces for each 'level':
-0.1 GPA penalty for any violations
- (Lack of necessary instructions may result in JavaScript functionality
marks getting missed in marking) as well as a style penalty
Submitting your work:
- The html documents for the three parts (and any local content such as
images or videos) of the assignment must be electronically submitted according to
the assignment submission requirements using
D2L. (Again you must
submit the web address of Part I in a Word document which also must be
submitted via D2L).
D2L configuration for this course
- You can (and really should) submit work as many times as you wish
before the due date
- Only your latest submission (what you submitted previously will be
overwritten by your latest submission)
- You can only submit one file per assignment. If you wish to submit
multiple files (not really needed for this assignment) then use 'zip' to
contain all the documents in one document: [How
to use zip in Windows 7]. Do not use other compression utilities
otherwise your submission may not be marked.
- You are not allowed to work in groups for this class. Copying
the work of another student will be regarded as academic misconduct
(cheating). For additional details about what is and is not okay for this
class please refer to the [notes
on misconduct for this course].
Points to keep in mind:
- Due time: All assignments are due at 4 PM on the
due dates
listed on the course web page. Late assignments or components of
assignments will not be accepted for marking without approval for an
extension beforehand. What you have submitted in D2L as of the due date is
what will be marked.
- Extensions may be granted for reasonable cases by the course
instructor with the receipt of the appropriate documentation (e.g., a
doctor's note). Typical examples of reasonable cases for an extension
include: illness or a death in the family. Cases where extensions will not
be granted include situations that are typical of student life: having
multiple due dates, work commitments etc. Tutorial instructors (TA's) will
not be able to provide extension on their own and must receive permission
from the course instructor first. (Note: Forgetting to hand your assignment
or a component of your assignment in does not constitute a sufficient reason
for handing your assignment late).
- Method of submission: You are to submit your assignment using D2L
[help
link]. Make sure that you [check
the contents of your submitted files] (e.g., is the file okay or was it
corrupted, is it the correct version etc.). It's your responsibility to do
this! (Make sure that your submit your assignment with enough time before it
comes due for you to do a check).
- Collaboration:
Assignments must reflect individual work, group work
is not allowed in this class nor can you copy the work of others. To avoid
problems students should not see each others assignment solution.
- Execution: programs must work on the browsers in the 203 computer
lab. It's up to you to test and check that this is the case. Non-functional
submissions will receive only partial credit (if any at all).