- Declares a 2D 5x5 array as an attribute. Each element refers to
an Entity object (1 mark)
|
- Defines a constructor which initializes each array element to 'null'. The element at [1][1] will refer to an
Entity
object (the starting location of the one entity in the simulation).
(2 marks: 1 for initializing whole array to null and 1 for
setting the starting location of the entity object)
|
- Using nested loops the simulated world will be displayed. The
appearance of an array element depends upon whether it is empty
(space character) or the one element that refers to an
Entity
object (the 'appearance'
attribute will be used - don't just hard code your output to the 'X'
or you won't get credit. Your program MUST be able to tell the
difference between a null element and a reference to an entity) (2 marks: 1 for each case)
|
- Each element is bound above, below, left and right by a line (see
Figure 1). (4 marks for every element properly bounded: 2
marks if bounded lines appear but (misses the top or bottom row) AS
WELL AS (missing the first or last column), 3 marks if bounded lines
appear but EITHER the top/bottom or far left/right is missed)
|
|
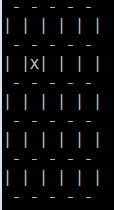
Figure 1: The display of array elements bounded in the four
directions (starting location of object at [1][1]) |
- Loop the display of the world until the user enters a negative
location for the row or column (next feature). (1 mark)
|
- (1) Prompts the user for the destination (row/column) to move the
entity. (2) A negative value for either coordinate will end the program
(regardless of what value was entered for the other coordinate e.g.,
(-1/-1), (-1,0), (20,-22) will all end the
program). (3) A value outside the bounds of the array will result in an
appropriate error message, non-negative out-of-bound values will
still allow the program to loop again as long as the other
coordinate is not negative. (3 marks: 1 for each feature)
|
|
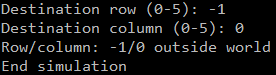
Figure 2: Any out-of-bound
destinations will result in an error message. Any negative coordinates
will end the simulation program. |
- (The previous feature must be implemented first). Assuming that
no out-of-bound coordinates have been entered, the program "moves" the entity to the
specifieddestination. The previous cell will appear 'empty' whereas the
destination will take on the appearance of the entity. (7 marks:
4 marks for destination cell, 3 marks for the source cell). Movement
must work for multiple turns in order to get credit (and not just
'apparently' work for one turn)
|