CPSC 233:
Assignment 4
Due March 9 at 4 PM
Introduction
Computer simulations are re-creation of a real-world
scenario. Simulations are important for many reasons including (but not limited
to) safety and cost. Also simulations may assist in decision making by allowing
alternatives to be tested before a course of action is chosen. For this
assignment you are to implement a simple race simulation (see Figure 1). One
competitor is controlled by the player ‘P’ the other is
computer-controlled ‘C’,
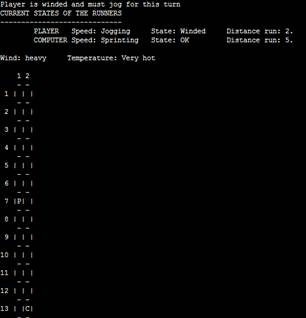 |
Figure 1: Race simulation |
Each runner will start at Row 1 and travel to Row 50. When one or more racers reach the last row the simulation will end
(Figure 2). If the player reaches row 50 first then the game is won. If the computer gets
there first then the game is lost. Tie games occur when both of them reach the
finish at the same time. Finally if the user quits the simulation prematurely
then the race is neither won nor lost. Because of the length of the track only a
part of it will be displayed at a time.
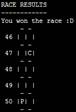 |
Figure 2: End of the race, at
least one runner reached the last row.
|
Each runner can run at one of three speeds: jog, run, sprint. The latter two
speeds results in more rows being traveled but they come at a risk. There is the
possibility when traveling at higher speeds that the runner becomes winded and
if so the runner must jog the next turn. When sprinting there is the additional
chance that the runner will burn out and can only jog thereafter. The
player can choose his/her running speed. The computer will have an even chance
at travelling at one of the three possible speeds.
Also if a runner is traveling faster than a jog, the runner may become distracted
("break disciple") and
slow to a jog. This slowing is different from becoming winded. Becoming winded
or burnt out is (for this simulation) determined by physical characteristics;
the runner's body has given out. Breaking discipline is attributed to mental not
physical reasons.
Finally this simulation includes the effect of weather. The weather will
consist of two properties: the wind speed and the temperature. Higher wind
speeds can reduce the amount of distance traveled (or even push
slower runners back!) Higher temperatures increase the chance of getting winded
or burnt out. Weather is highly volatile and can change each turn.
Required classes for this program
- UserInterface
- Mode
- RaceSim
- Runner
- Track
- Weather
UserInterface: similar to the previous assignment this class is
responsible for all input and output. e.g., displaying menus, getting user
input, determining if the user's selection was valid/determining which option
was selected.
Mode: Its sole purpose is to determine if the program is operating in
the debugging mode. By default the program will not be operating in debugging
mode.
public class
Mode
{
public
static boolean debug = false;
}
When the program is in debugging mode, messages about the
state of the program will be displayed e.g., the previous row before a move, the
running speed selected and the destination row. To get credit for implementing
the debug mode (Feature #8) the exact content of the messages is left
to your discretion but this mode should be implemented early on to aid in
testing/debugging. To speed up marking there are some features that must be
clearly illustrated as working via the debug mode in order for you to get
credit for that feature. These features will be marked as
("Debug
must show feature working"). Because of the unusual nature
of this class it can have a static implementation. No other classes
except for class Mode should be static (methods are static so an instance
doesn't get instantiated).
RaceSim: The 'Driver'
class for this program. The starting execution point that should contain a
reference to the user interface class:
public static
void main (String [] args) {
UserInterface
anInterface = new UserInterface ();
: :
}
Runner: the entities that travel along the race course.
Minimum required attributes: current running mode (jog, run, sprint), and
something to track if the runner is 'winded' or 'burnt out' (see Feature #7a & b).
Also each runner needs an 'appearance'
field, a single character that determines how the runner is represented (the
runner controlled by the user is a 'P'
while the computer player is a 'C').
Finally each runner should track its current location (row/column) on the race
track because the destination is going to be based on the current location.
These are just a few of the attributes needed for class Runner you will almost
certainly have more.
Minimum required methods: everything that modifies/retrieves the attributes
(there will be many). It should also include methods that are somehow related to
an instance even though they don't directly access or modify the attributes. For example determining if the runner gets distracted (see
Feature #7c) should be implemented in one or more methods of this class because
whether a particular runner gets distracted is determined for each runner,
therefore the functionality should be implemented as a method of class Runner.
Track: similar to the Manager class in the previous assignment it
tracks the core data used by the program. This program's Track class is
responsible for storing and managing all the information used in the simulated
world (the race track).
Minimum required attributes: the simulated world (a 2D array of references to
'Runner' objects):
private Runner
[][] raceCourse; Each element in the race course
array will either be null (signifying an empty location on the track) or refer
to a runner object.
Minimum required methods: all behaviors associated with managing the data
attributes (e.g., displaying the world, changing data in the world - moving
runners between locations, checking if the race is finished (when a runner
reaches the end).
Weather: There will only be one instance of this class for the whole
program. (This is not the same as a class consisting only of static methods!
This class should not have static methods.) This simulation tracks two aspects of the weather: the wind strength
and the current temperature. There are three possible wind strengths: no wind,
mild wind and heavy wind (1/3 chance of each) .There are four temperature
states: average, hot, very hot, heat wave (1/4 chance of each).
Program features (you will also be graded on other criteria such as
style and documentation as listed in the marking key): After creating the
above class definitions here are features that should be included in a
working program.
Note: the marking key for this assignment will be set up so you can get a
top grade without necessarily implementing each and every feature (there
will be a margin of error built in). However in order to prepare yourself
for the exam make sure you attempt (better yet complete) as many features as
possible.
- Displays an introduction to the program that describes the
rules (once as the program starts).
|
- Displays a sign off exit message when the program ends.
|
- Program runs until the end condition has occurred. If the
program can determine a win, lose or tie then the
status of race should be displayed at this time. Otherwise the
program will only end if the user quits the game (enters 'q' or
'Q') at the end of a turn. To make it easier for the user to see
the results the program should display the last few rows of the
track when the simulation ends (see Figure 2).
|
- Each turn a menu of options will be displayed that allows
the user to select the running speed of the human controlled
runner: (j)og, (r)un (s)print (q)uit
simulation.
|
- The track is displayed using a numbered grid. Because the
length of the track will exceed most screen displays the program
should pause the display the track part way through the display
(after one screen of data has been shown)
and wait for user input. To help your marker (and you) determine if features of
your program are working properly, as the program displays the
track it should also show the following information for each
runner (Figure 1):
|
|
- The running mode (jogging, running or sprinting).
- The distance to be traveled that turn
(determined by running speed minus any penalties due to the
wind).
- If the runner is okay, winded or burnt out.
|
- The current weather conditions (wind and temperature) are determined
at the start of each turn. Wind effects the number of squares that can be traveled by
each runner:
0
(no wind), -1 (mild wind) to -2 (heavy wind) movement. It's
possible that the wind can actually push a runner backwards (but
not back from the first row). Temperature will reduce the
runner's endurance, which can affect if a runner gets winded or
burns out: -0/no effect (average) +10%(hot), +20% (very hot),
+30% (heat wave). (Debug must show feature working)
|
- Runners can move at one of three speeds: jog (move 3 units),
run (move 1 - 6 units), sprint (move 3 - 12 units). The user
will be prompted for the speed of the
human-controlled runner. The program will
randomly determine which of the 3 speeds that the computer
controlled runner travel. (Debug must show
each of the below features working)
|
|
- Program checks if the runners traveling at a 'run' speed get
winded: There's 20% chance per turn a runner may get winded
and must (no option) switch to a jog the next turn, the
turn after that the runner may start running faster again. The
probability may be increased by higher temperatures (Feature
#6).
|
|
-
Program's check if runners traveling at a 'sprint' get winded or
burn out:
there's a 20% chance to get winded and switch to a jog for a turn,
20%
chance that the runner may burn out and be stuck at a jog for
the remainder of the race, 60% chance to maintain the sprint
that turn. The probability of getting winded or burning
out may be increased by higher temperatures (Feature #6).
|
|
- Program checks for distraction: There is a 25% possibility
that when the runner is traveling faster than a jog (whether
that speed was selected by the user or randomly generated by the
computer program) that the runner will become distracted, ignore
the command to run faster and just jog.
|
- Debug mode implemented: Debug mode can be toggled at the
start of each turn with a hidden menu option ('d' or 'D' instead
of selecting one of the listed options) to toggle debug mode. In order to get credit for
debug mode you must use debugging messages in some form. In
order to get credit for features labeled "Debug must show
feature working" debug mode must show enough information so
that the marker can quickly determine if the feature has been
implemented correctly. You can check with your marker for the
specifics on this but generally you are should show the value of
different variables in order to demonstrate that the feature
works. For example to show that your program increases the
sprint distance it might show the following information when
debug mode is engaged: base sprint distance (the randomly
generated value and the value after the modifications due to
wind. How many marks you receive for these features will be
determined by how well your debugging messages communicate the
necessary
information.
|
- Additional bonus feature: instead of displaying the
simulation in text, a graphical library (e.g., Java 2D, AWT,
Swing etc. is used). The interface itself can still be console
based (i.e., select a menu option via console input) just the
output is graphical. Your graphics don't have to be fancy
(e.g., you can use different shapes, color and/or text to
distinguish the runners) but the graphical version must clearly
represent the same information as the text-based equivalent
(e.g., don't use rectangles of the same size and appearance for
each runner). You won't get a lot of extra marks for
implementing this feature (because graphics isn't a key feature
of this program) and you will either have to be familiar with a
library or be willing to invest the time to learn one on your
own. But I did include this feature in case you wanted to spice
up your program and/or you wanted to increase the probability
that your assignment received a higher grade.
|
- Additional bonus feature: program adds sound effects. Your
program should sparingly add appropriate sound effects.
Similar to the previous feature, this feature won't be worth a
lot of marks and will require familiarity with classes to play
sound, or the willingness to learn how they work on your own.
|
Submitting your work:
- Assignments (source code/'dot-java' files) must be electronically
submitted according to [the
assignment submission requirements]. If there are any special
instructions needed to run your program, e.g., images needed, the
instructions should be included in the documentation for the Driver
class. You are to electronically submit the extra required files along
with your source code (make sure you don't forgot to submit all the
dot-Java files). There is no need to compress the files via zip or
another program.
- As a reminder, you are not allowed to work in groups for this class.
Copying the work of another student will
be regarded as academic misconduct (cheating). For additional details
about what is and is not okay for this class please refer to the
following [link].
- Before you submit your assignment to help make sure that you haven't
missed anything here is a [checklist] of items to be used in marking.
External libraries that can be used
- Libraries that allow for text-based (console) input and output.
- Classes used to generate random values such as Class Random or class
Math.
- (If the second last feature is implemented). Java libraries
that draw two-dimensional graphics.
- (If the last feature is implemented): Java libraries that play sound
files.