CPSC 233: Assignment 6
New concepts to be applied in this assignment
How to implement a graphical user interface
with Java (relevant classes)
- JFrames
- JLists
- Classes that handle layout (e.g., GridBagLayout)
- JTextfields
- JLabels
- JButtons
- Listener interfaces and classes (e.g., ones that implement ActionListener
or extend WindowAdaptor)
File-based input and output:
- FileReader
- BufferedReader
- FileWriter
- PrintWriter
Introduction
For this assignment you are to implement a
simple GUI (graphical user interface) that allows a person to manage a list of
email contacts.
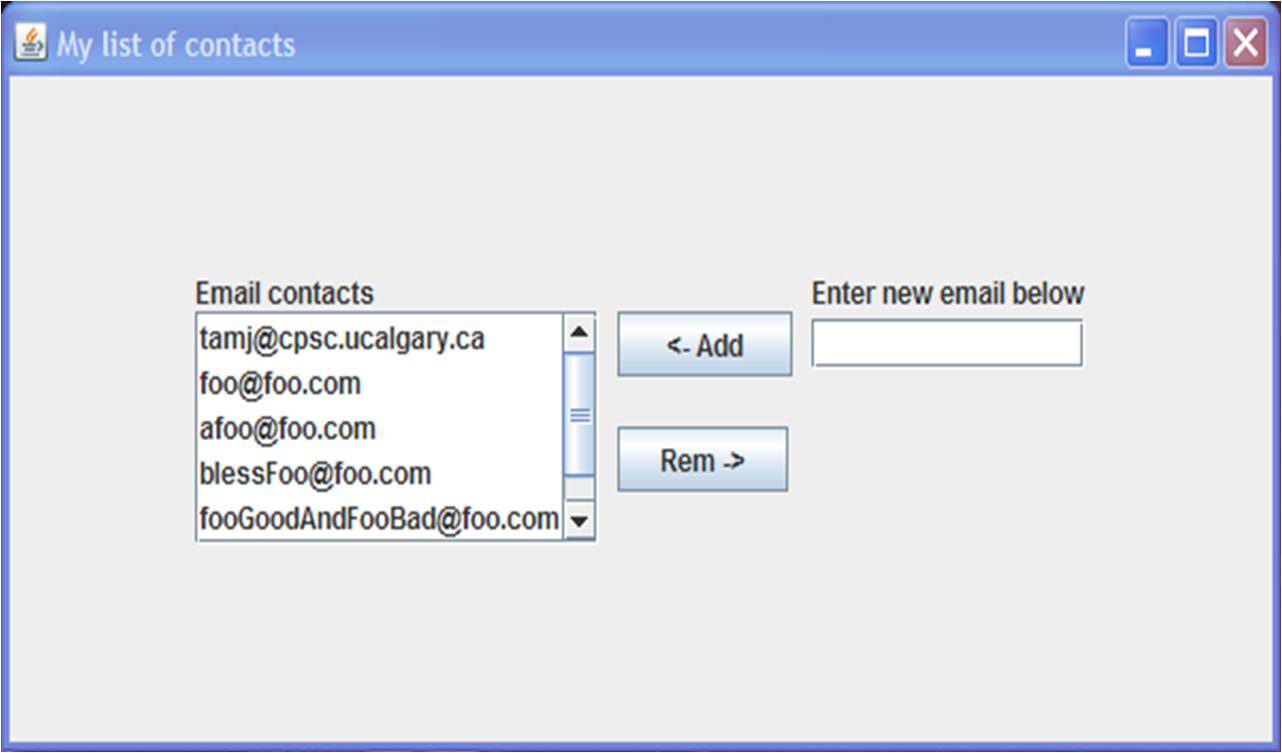
The information for the list of contacts will be automatically loaded from
file and added to the list as the program is run. The user can add new contacts
to the list or remove existing ones. The information for a new contact will be
entered in the input field (beside the add button). The program will provide
information to the user about it's state (e.g., when adding a new contact) or
when errors have occurred (e.g., a new contact will not be added when the input
field is empty). To simplify the marking of assignments make sure that you use
the exact file names specified below). When the user shuts down the
program by closing the window the updated information for the contacts will
automatically be saved to file.
Features
- When the program is run it will display the window which contains the
graphical controls (2 labels, 2 buttons, a list and a text field) and those
controls are laid out roughly the same fashion as shown in the picture.
- The program automatically loads the list of contacts from the file "contacts.txt"
when the program is run. A try-catch block must be used when a method is
called that can throw an exception.
- The program automatically saves the list of contacts to the file "updated_contacts.txt"
when the window is closed. A try-catch block must be used when a method is
called that can throw an exception.
- The user can remove a contact from the list by selecting the contact and
pressing the remove button.
- Text that is entered into the text field will be added to the end of the
list when the add button is pressed.
- Text that is entered into the text field will be added to the end of the
list when the cursor (focus) is in the text field and the user hits enter.
- The title bar of the frame indicates to the user when an existing contact
was removed (the remove feature must be implemented first). The message in the
title bar will remain for a few moments and then the text in the title bar
will revert back to what was originally there. Because you won't have learned
how to create threads, the interface will "lock" during this period of time
and will not accept any input from the user.
- In a fashion similar to the previous feature the title bar of the frame
will temporarily display a status message when a new contact is added to the
list (one or both of the add features must be implemented first).
- A status message will appear in the title bar when the close window
control is used to shut down the program.
- The program won't add an empty contact to the list (input field empty) and
in such cases it will display an error message in the title bar.
- One or more of the 'layout' classes are used to manage the layout and your
program roughly looks like the one in the picture.
Submitting your work:
- Assignments (source code/'dot-java' files and a short README file listing
which of the above features that you completed) must be electronically
submitted according to [the assignment
submission requirements].
- As a reminder, you are not allowed to work in groups for this class.
Copying the work of another student will be
regarded as academic misconduct (cheating). For additional details about what
is and is not okay for this class please refer to the following [link].
- Before you submit your assignment to help make sure that you haven't
missed anything here is a [checklist] of items to be used in marking.
Resource file
External libraries that can be used
- GUI widget classes for the graphical components.
- Listener classes and interfaces to react to events.
- Classes for file input and output (as well as the corresponding exception
classes).