CPSC 231: Assignment 5 (Due Friday June 13: Worth 6%)
A link to last summer's assignment 5 (The
Game of Life) that you may or may not find useful. You can also run the
source code for the solution to this assignment by looking under the path:
/home/231/assignments/assignment5/oldAssignment5.
The quest for the fountain of fulfillment!
This is an adventure game where the player is
given an overview (two-dimensional) of the game world. In the full version
of the game there are two locations that the player can explore: the town and a
dungeon. In each location there may be obstacles, such as trees and
buildings, to navigate around or there may be puzzles, such as locked doors, to
solve. In the full version of the game the player wins the game by finding
the legendary fountain of fulfillment (see Figure 0) which allows the allows the
player's every dream and goal to be satisfied and fulfilled.
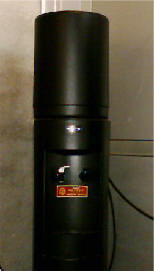
Figure 0: The legendary fountain of
fulfillment!
Assignment description
For this assignment you are to implement an
ASCII (text-only) version of "The quest for the fountain of fulfillment" game.
Each location (town and dungeon) is composed of a 10x10 grid of squares. A
square may be empty or it may contain an object. The game is played on a
turn-by-turn basis. During a turn the player is allowed the option of
moving onto another square or quitting the game. At the end of each turn
the map will be redrawn to update the map to reflect the changes that occurred
during that turn. The game continues on a turn-by-turn basis until either
the player wins the game or quits the program.
Location I: The town
The player starts off in the town level (see
Figure 1).
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
@ |
|
|
|
|
|
|
u |
# |
# |
|
|
b |
b |
|
|
|
X |
# |
# |
|
|
b |
b |
|
|
|
|
# |
# |
|
|
|
|
|
|
|
b |
# |
# |
u |
|
u |
u |
u |
u |
u |
u |
# |
# |
b |
|
|
|
|
|
|
|
# |
# |
|
|
|
|
b |
|
b |
|
# |
# |
|
|
|
b |
|
|
|
|
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure
1: Map of the town location
Each square in the grid for the town can
contain one and only one of the following items:
1. Town walls are represented by the
"#" character. The adventurer cannot enter a square containing a wall.
Because the town wall completely surrounds the entire town, it forms an
impenetrable barrier around the town.
2. Buildings are represented by the
"b" character. Buildings behave in a similar fashion to town walls in that
the adventurer cannot enter a square containing a building. The difference
is that buildings are not confined exclusively to the outer bounds of the town.
3. The adventurer is represented by
the "@" character. At the beginning of the game the adventurer starts off
near the top left hand corner of the map: 2nd row from the top, 2nd column from
the left (see Figure 1). The adventurer is the player's manifestation in
the game and how he or she explores the game world. The adventurer can
move in the four compass directions (north, south, east and west) or even pick
up objects (in the dungeon only). The orientation of the compass
directions on the map are shown in Figure 2.
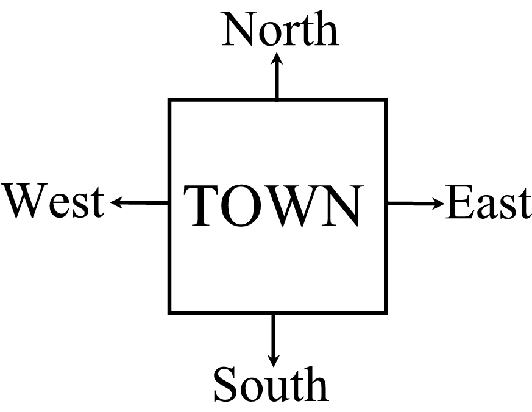
Figure 2: Compass directions
Each turn the player will be presented with a
series of menu options that he or she can select from (see Figure 3). The
player interacts with the game world by selecting one of the options in the
menu.
Movement options:
(N)orth
(W)est
(E)ast
(S)outh
(Q)uit program
|
Enter choice:
|
Figure 3: Menu options
As mentioned previously the adventurer can
move only one square per turn and only if the square that does not contain walls
and buildings (town) or walls and locked doors (dungeon). For
example, in Figure 4 we see that the adventurer is on the square near the top
left hand corner of the town.
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
|
|
@ |
|
|
|
|
u |
# |
# |
|
|
b |
b |
|
|
|
X |
# |
# |
|
|
b |
b |
|
|
|
|
# |
# |
|
|
|
|
|
|
|
b |
# |
# |
u |
|
u |
u |
u |
u |
u |
u |
# |
# |
b |
|
|
|
|
|
|
|
# |
# |
|
|
|
|
b |
|
b |
|
# |
# |
|
|
|
b |
|
|
|
|
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure
4: Movement options
The adventurer can normally move in the four
directions of the compass, however in this example the northern path is barred
by the wall while the southern path is blocked by a building. Thus the
only valid directions of movement in this case are west and east. If the
player selects a direction that is blocked then the adventurer will not move and
the program will display an error message which indicates that this square
cannot be entered and why e.g., "Cannot enter the square because it contains a
building". The program will then continue presenting the menu of options
shown in Figure 3 until the player selects a valid square or quits the game.
4. Bushes are represented by the "u"
character. The adventurer can move onto a square containing a bush.
In figure 5A we see the adventurer before the move.
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
|
|
|
|
|
|
@ |
u |
# |
# |
|
|
b |
b |
|
|
|
X |
# |
# |
|
|
b |
b |
|
|
|
|
# |
# |
|
|
|
|
|
|
|
b |
# |
# |
u |
|
u |
u |
u |
u |
u |
u |
# |
# |
b |
|
|
|
|
|
|
|
# |
# |
|
|
|
|
b |
|
b |
|
# |
# |
|
|
|
b |
|
|
|
|
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure
5A: Before move
If the player chooses to move east then the
"u" will changed to the "@" character indicating that the person has walked onto
top of the bush (Figure 5B).
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
|
|
|
|
|
|
|
@ |
# |
# |
|
|
b |
b |
|
|
|
X |
# |
# |
|
|
b |
b |
|
|
|
|
# |
# |
|
|
|
|
|
|
|
b |
# |
# |
u |
|
u |
u |
u |
u |
u |
u |
# |
# |
b |
|
|
|
|
|
|
|
# |
# |
|
|
|
|
b |
|
b |
|
# |
# |
|
|
|
b |
|
|
|
|
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure
5B: After the move
The bush however is now "crushed" so that
when the player moves off the square where the bush was located that square will
be shown as an empty square (Figure 5C).
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
|
|
|
|
|
|
@ |
|
# |
# |
|
|
b |
b |
|
|
|
X |
# |
# |
|
|
b |
b |
|
|
|
|
# |
# |
|
|
|
|
|
|
|
b |
# |
# |
u |
|
u |
u |
u |
u |
u |
u |
# |
# |
b |
|
|
|
|
|
|
|
# |
# |
|
|
|
|
b |
|
b |
|
# |
# |
|
|
|
b |
|
|
|
|
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure
5C: The bush has been crushed
5. The dungeon entrance
is represented with the "X" character. When the adventurer is on a square
horizontally or vertically adjacent to the dungeon entrance (Figure 6A) then the
player can choose to enter the dungeon by moving the adventurer onto that
square.
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
b |
b |
|
|
|
X |
# |
# |
|
|
b |
b |
|
|
|
@ |
# |
# |
|
|
|
|
|
|
|
b |
# |
# |
u |
|
u |
u |
u |
u |
u |
u |
# |
# |
b |
|
|
|
|
|
|
|
# |
# |
|
|
|
|
b |
|
b |
|
# |
# |
|
|
|
b |
|
|
|
|
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure
6: Next to the entrance to the dungeon
When the player moves onto the square
containing the dungeon entrance then the adventurer will be transported to the
dungeon. A message will appear onscreen indicating that the adventurer is
now entering the dungeon. The program will no longer draw the grid
for the town but instead the grid for the dungeon will be shown (Figure 7).
Location II: The dungeon
The player can only enter the dungeon level
through the entrance in the town (described in part 5 of the previous section).
Interaction with the game in the dungeon level is very similar to the town
level. What is different is the objects that can be found here (Figure 7):
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
t |
# |
|
|
|
|
|
|
# |
# |
k |
# |
|
|
|
|
|
X |
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
# |
# |
# |
# |
# |
|
|
|
|
|
d |
|
f |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure 7: The dungeon
1. Walls
behave in a similar fashion here, the adventurer cannot move onto a square that
contains a wall. However, unlike in the town, the walls of the dungeon are
not restricted to the outer edges of the map.
2. The town entrance
is also depicted by an "X". When the character initially arrives in the
dungeon from the town the square that normally contains the entrance will not
contain the "X" but instead contain the character used to represent the
adventurer "@" as shown in Figure 8A. If the adventurer moves off
the square containing the entrance and then moves back onto it then the person
will travel back to the town. A message will appear onscreen indicating
that the adventurer is now entering the town and the grid for the town will be
displayed rather than the grid for the dungeon.
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
t |
# |
|
|
|
|
|
|
# |
# |
k |
# |
|
|
|
|
|
@ |
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
# |
# |
# |
# |
# |
|
|
|
|
|
d |
|
f |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure 8A: The adventurer first arrives in
the dungeon
Whenever the adventurer moves
off this square then the town entrance can be seen (Figure 8B).
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
t |
# |
|
|
|
|
|
|
# |
# |
k |
# |
|
|
|
|
@ |
X |
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
# |
# |
# |
# |
# |
|
|
|
|
|
d |
|
f |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure 8B: The adventurer makes the first
move in the dungeon
3. The Adventurer
can be controlled in the same fashion in the dungeon as in the town.
Squares that cannot be entered are ones that contain a wall or a locked door
(see #4 below). There are three special squares that are unique to
the dungeon: the key (#5), the teleporter square (#6), and the fountain of
fulfillment (#7).
4. The locked door
is represented with the "d" character. Normally the adventurer cannot
enter this square. However if the adventurer is carrying the key (#5) then
the door unlocks. The door disappears permanently from the dungeon and the
player can now move the adventurer onto this square. The message
indicating that the adventurer is carrying the key will no longer appear at this
point (see the point below).
5. The key
is represented by the "k" character. When the adventurer is on a
vertically or horizontally adjacent square to the key (Figure 9A) and is then
moved onto the square containing the key then a number of things occur.
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
t |
# |
|
|
|
|
|
|
# |
# |
k |
# |
|
|
|
|
|
X |
# |
# |
@ |
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
# |
# |
# |
# |
# |
|
|
|
|
|
d |
|
f |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure 9A: The adventurer can pick up the
key
The key character is replaced by the
character representing the adventurer. A message will now be displayed
below the map of the dungeon indicating that the adventurer now carries the key
and the key disappears from the dungeon (Figure 9B).
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
t |
# |
|
|
|
|
|
|
# |
# |
@ |
# |
|
|
|
|
|
X |
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
# |
# |
# |
# |
# |
|
|
|
|
|
d |
|
f |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
The adventurer now has the key |
Figure 9B: The key is picked up by the
adventurer
The latter part means that even after the
adventurer moves off square containing the key then that square will now be
empty (Figure 9C).
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
t |
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
X |
# |
# |
@ |
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
# |
# |
# |
# |
# |
|
|
|
|
|
d |
|
f |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure 9C: The key is no longer in the
dungeon
6. The teleporter square
is represented by the "t" character. When the player moves the adventurer
onto this square the adventurer will be randomly teleported onto one of the
unoccupied squares in the dungeon. For example in Figure 10A the
adventurer is standing next to the teleporter square.
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
t |
# |
|
|
|
|
|
|
# |
# |
@ |
# |
|
|
|
|
|
X |
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
# |
# |
# |
# |
# |
|
|
|
|
|
d |
|
f |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure 10A: The adventurer is ready for
teleportation
When the player moves the adventurer onto the square containing the
teleporter then the program will display an onscreen message indicating that the
adventurer is being teleported. The adventurer will then be randomly
placed in one of the empty square in the dungeon (Figure 10B shows an example).
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
t |
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
X |
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
@ |
|
|
|
# |
# |
# |
# |
# |
|
|
|
|
|
d |
|
f |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure 10B: The adventurer is now someplace
else
7. The fountain of fulfillment
is represented by the "f" character and is the object of your quest. When
the adventurer is moved onto to the square that is adjacent to the fountain then
the player has won the game (Figure 11). At this point a suitable
congratulatory message is displayed onscreen and the program ends.
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
t |
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
X |
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
# |
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
|
|
|
# |
# |
|
|
|
|
|
# |
# |
# |
# |
# |
|
|
|
|
|
|
@ |
f |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
# |
Figure 11: You win!
Grading
Base assignment: C+
-
Game displays the town
-
A menu of options is displayed to the player
(all five menu options work)
-
Adventurer is displayed onscreen and can move
in the four directions
-
Town contains the following objects
-
Wall around town: can't enter this square
-
Buildings: can't enter this square
- Bushes: can be crushed by the adventurer
Extra features: Implementing each one will increase your grade by one letter
"step" (e.g., "B" to "B+")
-
An entrance to the dungeon is added to the
town level. Adventurers can travel to and from the dungeon via the
entrance. The game works in the same way in dungeon that it works in the
town. The dungeon contains: an entrance to the town and a locked door.
Feature #1 must obviously be implemented before credit will be given for
features #2 - 4.
-
The dungeon contains a key that can be picked
up and will unlock the locked door. This feature must be implemented
before credit will be given for feature #4 (because the adventurer has to
pass through the locked door in order to reach the fountain).
-
The dungeon contains a teleporter.
-
The player can win the game by moving to the
square next to the fountain of fulfillment.
Implementing the assignment using modular design: Your assignment grade can
increase by two letter "steps" (e.g., "B" to "A-")
You will receive credit for implementing your program in a modular fashion
if it is broken down into functions and procedures.
Other submission requirements
-
Good coding style and documentation:
They will play a role in determining your final
grade for this assignment. Your grade can be reduced by a letter step e.g., "A"
to "A-" for poor programming style.
-
Method of submission. Due to the nature
of this particular assignment you will not be handing in a typescript.
Instead you will print out your source code (via lpr) and hand that into the
drop box. To demonstrate to your TA how your program works you must book a
time during the lab the following week. This is important! If you
neglect this part then your marker can only mark the assignment based on what
she receives.
New Concepts to be applied for the assignment
- Functions/procedures
- Two-dimensional arrays