CPSC 231: Assignment 5 (Worth 4%) Monday June 7
New Concepts to be applied for the assignment
-
Problem decomposition through the use of
functions and procedures
-
One dimensional arrays
Writing a grade management program
For this assignment you are to write a
program that allows a course instructor to track and manage a list of grades for
a small class. For the full version the program will allow the user to:
enter a set of grades for the class, to display the class list and some
statistics about the performance of the class as a whole.
Grading
Assignments that receive a "C"
The program will allow the user to enter in
the grades for a class with a maximum size of 25 students. The list of
grades will be stored in two one-dimensional arrays: one array will store the
percentage value, the other grade will store the corresponding letter that the
percentage corresponds to.
Mapping of percentages to letter grades
Range |
Letter |
percentage >= 90 |
A |
80 >= percentage < 90 |
B |
70 >= percentage < 80 |
C |
60 >= percentage < 70 |
D |
percentage < 60 |
F |
The user can enter the
enter the grades for smaller class size by entering a negative value for a
grade. The negative value is not counted
as one of the grades. You must write a function or procedure that will allow the
user to enter the grades. You will then write another procedure that will
display the list of grades. If there are fewer than 25 students in the
class then the number of grades that the program displays will match the current
class size (see the sample output below).
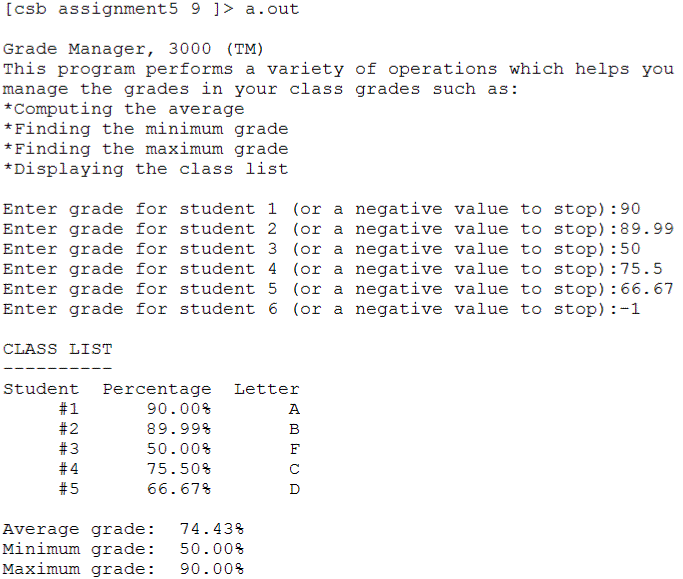
As shown above, the output of the program must
be formatted into three columns that are right-aligned. Percentage values
are displayed with a maximum of two decimal places of precision. Also if the class is empty (i.e., it has no
students in it) then the program will indicate that the class is empty rather
than displaying an empty list. Assignment submissions must follow also
follow good coding style and must be fully documented.

It is okay if you wish to write additional functions or procedures (besides
the two listed above) in order to complete your assignment, in fact you will
probably find it essential. How you wish to structure the remainder of
your program is up to you, just make sure that your solution is guided by good
design principles e.g., avoid putting all the code into a single function or
procedure (especially the main procedure), each function or procedure should
have a well defined task that gives it a purpose.
Additional capabilities
Implementing features #1 - 3 will yield a
grade increase of two "steps" (e.g., "C" to "B-"). Implementing feature
number #4 will yield a grade increase of one step. These extra features
can be implemented in any order and in any combination that you wish.
Implementing all four features will yield a grade increase of seven steps ("C" to
"A+").
-
Compute the average grade: Implement a
function or procedure that will determine the average grade for the class.
For non-empty classes the average grade will be also be shown when the display
procedure is called.
-
Find the minimum grade: Implement a function
or procedure that will find the lowest grade in the class. For non-empty
classes the minimum grade will be also be shown when the display procedure is
called.
-
Find the maximum grade: Implement a
function or procedure that will find the highest grade in the class. For
non-empty classes the maximum grade will be also be shown when the display
procedure is called.
- Increase the class size from 25 students to
50 students. In order for you to
receive credit for this extra feature you must hand in two versions of your
assignment, one with a maximum class size of 25 students and one with a
maximum class size of 50 students. Hint: There is a hard way of doing
this an and easy way. Think back the lecture when I first talked about writing
programs and good style for a clue as to what the easy way is.
Other submission requirements
-
Good coding style and documentation:
They will play a role in determining your final
grade for this assignment. Your grade can be reduced by a letter step
or more if your submission was implemented using bad style conventions (e.g., "A" to "A-"
for employing poor naming
conventions for identifiers, insufficient documentation, the use of global
variables or non-modular design).
-
Include a README file in your submission: For this assignment
your README file must indicate what grade level has been completed (e.g., A, B or C).
This will allow your marker to quickly determine what he or she must look for
and speed up the marking process.
-
Assignments (source code and the README file) must be electronically
submitted via submit.
In addition a paper print out of the source code and README must be handed into the
assignment drop box for the lab that you are registered in (located on the
second floor of the Math Sciences building). Electronically submitting the
assignment allows your marker to run the code in order to quickly determine what
features were implemented. Providing a paper printout makes it easier for
your marker to read and flip through your source code.
Sample Executable
You can run a sample executable called "grades"
which can be found in Unix in the directory: /home/231/assignments/assignment5